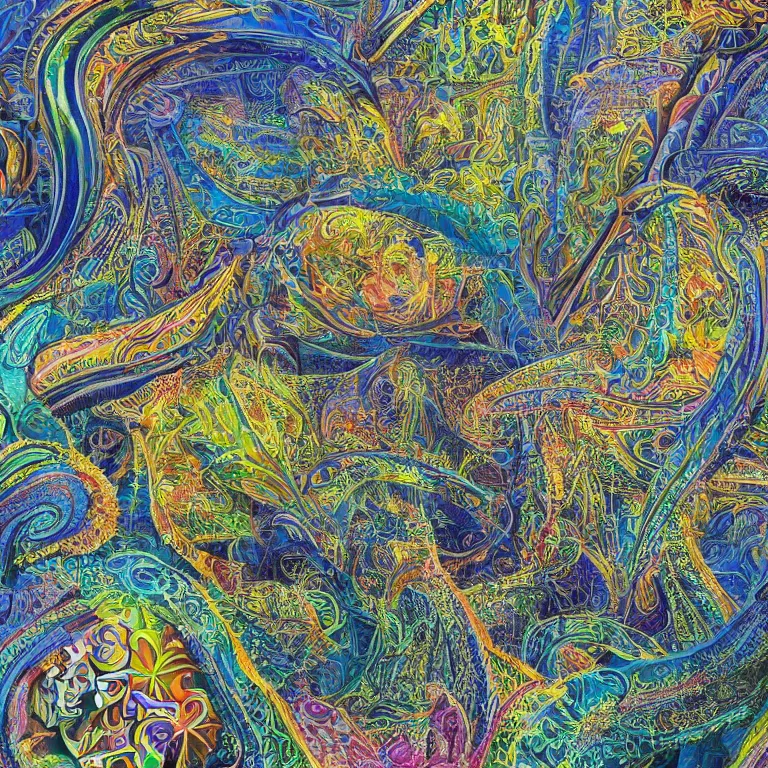
Jan 30th, 2023
pdb
Module
Python provides a built-in module called pdb
which stands for Python Debugger.
This module allows you to interactively debug your Python code by providing an interactive prompt and the ability to step through your code line by line.
In this post, we will take a look at some of the most commonly used methods of the pdb
module and how they can be used to debug your Python code.
I've heard complaints about the lack of debugging tools for Python, including the inability to run programs line by line with breakpoints, and to see the values of variables during runtime, but the pdb
module allows programmers to do all of these.
The pdb
module provides a number of different methods that can be used to debug your code.
The most basic method is the run()
method, which starts the interactive prompt and runs the code until a breakpoint is hit.
Here's an example of how to use the run()
method:
import pdb def add_numbers(a, b): result = a + b return result pdb.run("add_numbers(1, 2)")
In this example, we import the pdb
module, define a simple function add_numbers
that takes two arguments and returns the sum of them.
We then use the run()
method to run the function and start the interactive prompt.
Once the function is run, you will see the (Pdb) prompt where you can use the command n
to go to next line, c
to continue until the next breakpoint and others.
Another useful method provided by the pdb
module is the runcall()
method.
This method runs a function and starts the interactive prompt when the function is called.
Here's an example of how to use the runcall()
method:
import pdb def add_numbers(a, b): result = a + b return result pdb.runcall(add_numbers, 1, 2)
In this example, we use the runcall()
method to run the add_numbers function with arguments 1 and 2, and start the interactive prompt when the function is called.
Another method provided by the pdb
module is the set_trace()
method, it allows you to set a breakpoint in your code at the point where you call this method.
Here's an example of how to use the set_trace()
method:
import pdb def add_numbers(a, b): pdb.set_trace() result = a + b return result add_numbers(1, 2)
In this example, we use the set_trace()
method to set a breakpoint in the add_numbers
function at the point where the method is called, and start the interactive prompt.
In summary, the pdb
module in Python provides a way to interactively debug your code by providing an interactive prompt and the ability to step through your code line by line.
The pdb
module provides a number of different methods, such as run()
, runcall()
and set_trace()
which can be used to debug your code in different ways.
By using these methods, you can easily identify and fix bugs in your code.
It's worth noting that while using the pdb
module is not required in Python, it is a powerful tool that can greatly improve your debugging process.
The interactive prompt provided by the pdb
module allows you to easily navigate through your code and inspect the values of variables, making it easier to identify and fix bugs.
Additionally, the ability to step through your code line by line allows you to easily trace the execution of your code and identify the cause of the bugs.
In conclusion, the pdb
module in Python is a powerful tool that allows you to interactively debug your code by providing an interactive prompt and the ability to step through your code line by line. Understanding and using the pdb
module is an essential part of creating high-quality Python code and can greatly improve your debugging process.