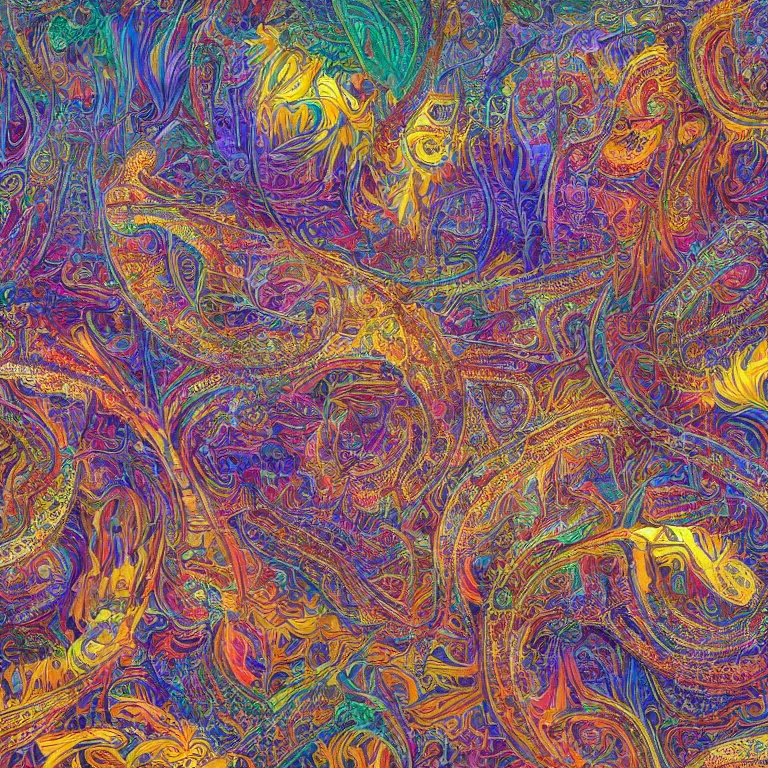
Jan 29th, 2023
The typing
module in Python is a module for working with type hints.
Type hints are a way to indicate the type of a variable, function, or method in Python, allowing for improved code readability, better error checking and more efficient code execution.
They also allow you, the programmer, to gain a better insight on how datatyping works in languages, and this skill transfers over to most other programming languages as well.
In this post, we will take a look at some of the most commonly used methods of the typing
module and how they can be used to improve the quality of your code.
The typing
module provides a number of different types that can be used to indicate the type of a variable, function, or method.
For example, the List
type can be used to indicate that a variable is a list of a specific type of elements.
Here's an example of how to use the List
type:
from typing import List def add_numbers(numbers: List[int]) -> int: return sum(numbers) print(add_numbers([1, 2, 3, 4, 5])) # Output: 15 print(add_numbers([1, 2, '3', 4, 5])) # Output: TypeError
In this example, we use the List
type to indicate that the numbers
argument of the add_numbers
function is a list of integers.
We also use the ->
syntax to indicate that the function returns an integer.
If we try to pass a list that contains elements of a different type to the function, we'll get a TypeError
.
Another useful type provided by the typing
module is the Tuple
type.
This type can be used to indicate that a variable is a tuple of specific types.
Here's an example of how to use the Tuple
type:
from typing import Tuple def add_numbers(numbers: Tuple[int, int]) -> int: return sum(numbers) print(add_numbers((1, 2))) # Output: 3 print(add_numbers((1, '2'))) # Output: TypeError
In this example, we use the Tuple
type to indicate that the numbers
argument of the add_numbers
function is a tuple of two integers.
If we try to pass a tuple that contains elements of a different type to the function, we'll get a TypeError
.
Another type provided by the typing
module is the Union
type.
It allows you to indicate that a variable can be of one of multiple types.
Here's an example of how to use the Union
type:
from typing import Union def add(a: Union[int,float], b: Union[int,float])-> Union[int,float]: return a+b print(add(1, 2)) # Output: 3 print(add(1.1, 2)) # Output: 3.1
In this example, we use the Union
type to indicate that the a
and b
arguments of the add
function can be of type int
or float
and the function will return the same type.
In summary, the typing
module in Python provides a way to improve the quality of your code by indicating the type of a variable, function, or method.
This allows for improved code readability, better error checking, and more efficient code execution.
The typing
module provides a number of different types, such as List
, Tuple
, Union
and many others, that can be used to indicate the type of a variable, function or method.
By using these types, you can ensure that your code is more robust and less prone to errors.
It's worth noting that while using type hints is not required in Python, it is a best practice to use them. Type hints make your code more readable and self-documenting, making it easier for others to understand and maintain your code. Additionally, using type hints can also improve the performance of your code, by enabling the use of type-specific optimization techniques.
In conclusion, the typing
module in Python is a powerful tool that allows you to improve the quality of your code by indicating the type of a variable, function, or method.
By using type hints, you can make your code more robust, readable, and efficient.
Understanding and using the typing
module is an essential part of creating high-quality Python code.