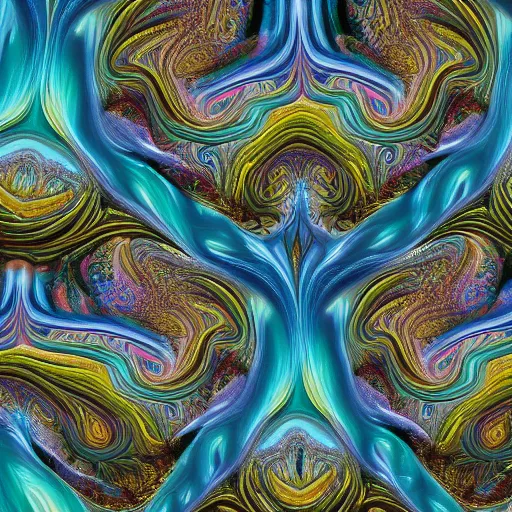
Jan 28th, 2023
Python provides two built-in modules, multiprocessing
and threading
, for working with multiple processes and threads.
Both modules allow you to create multiple concurrent execution threads to improve the performance of your Python program.
In this post, we'll take a look at some of the most commonly used methods of these modules and how they can be used to improve the performance of your program.
Separate Memory Processes with the Multiprocessing Module
The multiprocessing
module allows you to create multiple processes that run concurrently in separate memory spaces.
This is useful for parallelizing computationally intensive tasks that do not need to share memory.
Here's an example of how to use the Pool
class of the multiprocessing
module to parallelize a function:
from multiprocessing import Pool def square(x): return x*x # Create a pool of 4 worker processes with Pool(4) as p: # Apply the square function to each element of the input list result = p.map(square, [1, 2, 3, 4, 5]) print(result) # Output: [1, 4, 9, 16, 25]
In this example, we first import the Pool
class from the multiprocessing
module.
Then, we define a simple function square
that takes a single argument and returns its square.
We create a Pool
object with 4 worker processes, and then use the map()
method to apply the square function to each element of the input list.
The map()
method returns the result of the function applied to each element of the input list.
Shared Memory Threads with the Threading Module
The threading
module allows you to create multiple threads that run concurrently in the same memory space.
This is useful for parallelizing tasks that need to share memory.
Here's an example of how to use the Thread
class of the threading
module to parallelize a function:
from threading import Thread def square(x): return x*x # Create a list of input values input_list = [1, 2, 3, 4, 5] # Create a list to store the results result = [] # Create a new thread for each input value threads = [Thread(target=square, args=(x, result)) for x in input_list] # Start all threads for thread in threads: thread.start() # Wait for all threads to complete for thread in threads: thread.join() print(result) # Output: [1, 4, 9, 16, 25]
In this example, we first import the Thread
class from the threading
module.
Then, we define a simple function square
that takes a single argument and returns its square.
We create a Thread
object for each input value and start all threads.
The target
attribute is set to the function and the args
attribute is set to the input value and the results list.
We use the join()
method to wait for all threads to complete before printing the results.
In summary, the multiprocessing
and threading
modules provide a way to improve the performance of your Python program by parallelizing computationally intensive tasks.
The multiprocessing
module allows you to create multiple processes that run concurrently in separate memory spaces, while the threading
module allows you to create multiple threads that run concurrently in the same memory space.
It's important to note that while both modules can be used to improve performance, they are suited for different types of tasks.
The multiprocessing
module is better suited for tasks that do not need to share memory, while the threading
module is better suited for tasks that need to share memory.
When choosing between the multiprocessing
and threading
modules, it's important to consider the specific requirements of your task and the resources of your system.
The multiprocessing
module can be more efficient on systems with multiple cores or processors, as it can take full advantage of the additional resources.
On the other hand, the threading
module is more lightweight and can be a better choice for tasks that do not require a lot of computational power.
In conclusion, the multiprocessing
and threading
modules are powerful tools in Python that allow you to improve the performance of your program by parallelizing computationally intensive tasks.
Understanding and using these modules can greatly enhance the functionality and performance of your Python code.