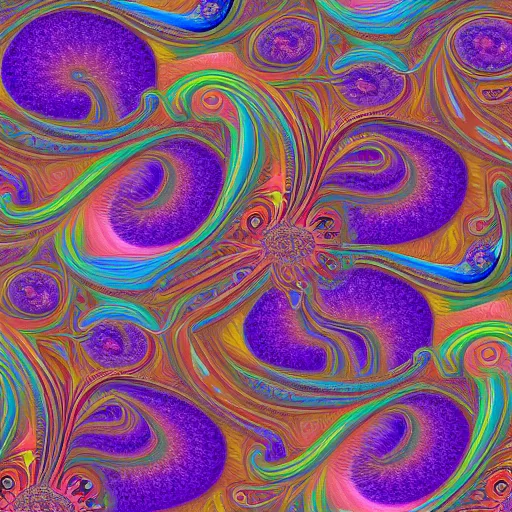
Jan 27th, 2023
In Python, dunder methods, also known as "magic" or "special" methods, are used to define how objects of a class behave in certain situations.
These methods are defined by double underscores at the beginning and end of their names (e.g. __init__()
, __add__()
).
They allow you to customize the behavior of built-in operations (e.g. addition, comparison, iteration) and provide a way to make your classes more Pythonic, and are similar to built-in methods of classes in other object oriented programming languages like C# and Java
In this post, we'll take a look at some of the most commonly used dunder methods and how they can be used in your classes.
One of the most commonly used dunder methods is the __init__()
method.
This method is used to initialize an object when it is created.
Here's an example of how to use it:
class MyClass: def __init__(self, value): self.value = value # Create an object of MyClass obj = MyClass(5) # Print the value attribute of the object print(obj.value) # Output: 5
In this example, we define a class MyClass with an __init__()
method that takes one argument, value, and assigns it to an attribute of the same name.
This is the same as a constructor like in other programming languages like C#/
Another commonly used dunder method is the __str__()
method.
This method is used to define how an object should be represented as a string.
Here's an example of how to use it:
class MyClass: def __init__(self, value): self.value = value def __str__(self): return 'MyClass object with value: {}'.format(self.value) # Create an object of MyClass obj = MyClass(5) # Print the object print(obj) # Output: 'MyClass object with value: 5'
In this example, we've defined the __str__()
method to return a string that includes the value attribute of the object.
This allows us to print the object in a more readable format.
Another useful dunder method is the __add__()
method.
This method is used to define how two objects of a class should be added together.
Here's an example of how to use it:
class MyClass: def __init__(self, value): self.value = value def __add__(self, other): return MyClass(self.value + other.value) # Create two objects of MyClass obj1 = MyClass(5) obj2 = MyClass(10) # Add the two objects result = obj1 + obj2 # Print the value attribute of the result object print(result.value) # Output: 15
In this example, we've defined the __add__()
method to create a new object with the sum of the value attributes of the two objects being added.
This allows us to use the + operator to add two objects of our class together.
Another dunder method is the __eq__()
method.
This method is used to define how two objects of a class should be compared for equality.
Here's an example of how to use it:
class MyClass: def __init__(self, value): self.value = value def __eq__(self, other): return self.value == other.value # Create two objects of MyClass obj1 = MyClass(5) obj2 = MyClass(5) obj3 = MyClass(10) # Compare the two objects print(obj1 == obj2) # Output: True print(obj1 == obj3) # Output: False
In this example, we've defined the __eq__()
method to compare the value attributes of the two objects being compared.
This allows us to use the == operator to compare two objects of our class.
The __len__()
method is used to define the length of an object.
Here's an example of how to use it:
class MyClass: def __init__(self, value): self.value = value def __len__(self): return len(self.value) # Create an object of MyClass obj = MyClass([1, 2, 3, 4, 5]) # Get the length of the object print(len(obj)) # Output: 5
In this example, we've defined the __len__()
method to return the length of the value attribute of the object.
This allows us to use the len() function to get the length of an object of our class.
The __iter__()
method is used to define an iterator for an object. Here's an example of how to use it:
class MyClass: def __init__(self, value): self.value = value self.index = 0 def __iter__(self): return self def __next__(self): if self.index >= len(self.value): raise StopIteration result = self.value[self.index] self.index += 1 return result # Create an object of MyClass obj = MyClass([1, 2, 3, 4, 5]) # Iterate over the object for item in obj: print(item) # Output: 1, 2, 3, 4, 5
In this example, we've defined the __iter__()
method to return the object itself and the __next__()
method to return the next item of the value attribute of the object.
This allows us to use the for loop to iterate over an object of our class.
As you can see, dunder methods in Python provide a powerful way to customize the behavior of built-in operations and make your classes more Pythonic. They allow you to define how objects of your class should behave in certain situations, making your code more readable, maintainable, and efficient.
It is important to note that while using dunder methods is not required, they can greatly enhance the functionality of your classes and make them more intuitive to work with.
For example, by implementing the __eq__()
method, you can ensure that two objects of your class can be compared for equality using the == operator, which makes it more readable and maintainable.
Similarly, by implementing the __len__()
method, you can ensure that the len() function can be used to get the length of an object of your class, which makes it more intuitive to work with.
It's also worth noting that not all dunder methods need to be implemented. Depending on the class you're creating, you may only need to implement a few of them. However, it's a good idea to be familiar with the available dunder methods so you can use them when they're appropriate.
In summary, dunder methods are an important part of creating well-designed and well-behaved classes in Python. They allow you to customize the behavior of built-in operations, making your classes more Pythonic and providing a way to make your code more readable, maintainable, and efficient. Understanding and using dunder methods is essential for creating high-quality Python code.