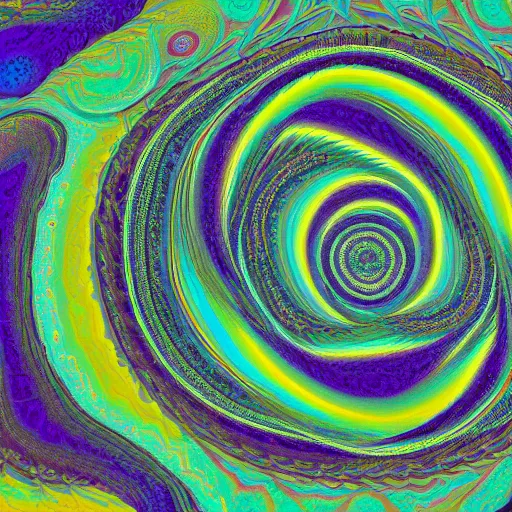
Jan 26th, 2023
The os module in Python provides a way for your Python program to interact with the underlying operating system. It provides a wide range of functionality for working with the file system, such as creating, deleting, and moving files and directories, as well as interacting with the environment variables and running command-line commands. In this post, we'll take a look at some of the most commonly used methods of the os module.
One of the most commonly used methods of the os module is the mkdir()
method.
This method is used to create a new directory. Here's an example of how to use it:
import os # Create a new directory os.mkdir('new_directory')
In this example, we first import the os module.
Then, we use the mkdir()
method to create a new directory called "new_directory".
Another useful method of the os module is the rename()
method.
This method is used to rename a file or directory. Here's an example of how to use it:
import os # Rename a file os.rename('old_file.txt', 'new_file.txt')
In this example, we first import the os module. Then, we use the rename()
method to rename the file "old_file.txt" to "new_file.txt".
The remove()
method is used to delete a file.
Here's an example of how to use it:
import os # Delete a file os.remove('file_to_delete.txt')
In this example, we first import the os module.
Then, we use the remove()
method to delete the file "file_to_delete.txt".
The chdir()
method is used to change the current working directory. Here's an example of how to use it:
import os # change the current working directory os.chdir('/path/to/directory')
In this example, we first import the os module.
Then, we use the chdir()
method to change the current working directory to "/path/to/directory".
The getcwd()
method is used to get the current working directory.
Here's an example of how to use it:
import os # get the current working directory current_directory = os.getcwd() print(current_directory)
In this example, we first import the os module.
Then, we use the getcwd()
method to get the current working directory and print it.
The os module also provides other methods and attributes, such as listdir()
, environ
, system()
, path
, and stat()
that can be used to interact with the file system and the environment in more advanced ways.
In conclusion, the os module is a powerful module in Python that provides a wide range of functionality for working with the file system, such as creating, deleting, and moving files and directories, as well as interacting with the environment variables and running command-line commands. It is a useful tool for developers to have in their toolkit when working on scripts that need to interact with the operating system.