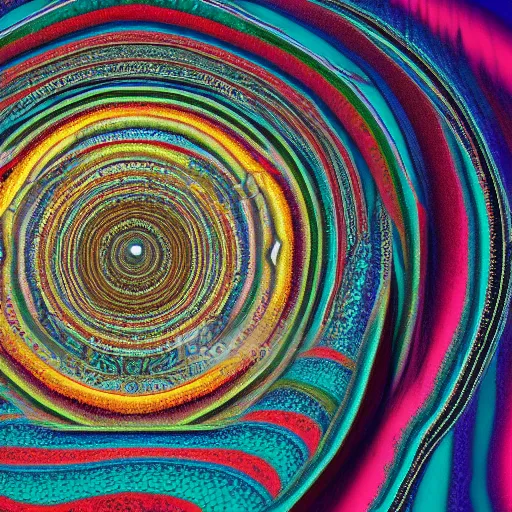
Jan 25th, 2023
The sys module in Python provides access to various system-specific parameters and functions.
It provides a way for your Python program to interact with the underlying operating system, such as interacting with command line arguments and controlling the interpreter's behavior.
One of the most commonly used attributes of the sys module is the argv
attribute.
This attribute is a list that contains the command-line arguments passed to a Python script.
These arguments can be accessed and processed within the script to provide additional functionality or to control the script's behavior based on the arguments passed.
The exit()
function is another useful method provided by the sys module.
This function is used to exit the interpreter by raising a SystemExit
.
The optional argument passed to the function is used as the return code for the script, this can be used to indicate the success or failure of the script.
The version
attribute returns a string that contains the version of the Python interpreter, this can be useful for version control and compatibility issues.
The path
attribute contains a list of strings that specifies the search path for modules, this can be manipulated to include new paths for importing modules.
This attribute is extremely useful when working with multiple projects and libraries.
The sys module also provides some other useful methods and attributes, such as stdin
, stdout
, stderr
, platform
, and exc_info()
that can be used to interact with the interpreter's input and output streams and to get information about the current exception.
The sys module can be considered as a Swiss Army knife for developers, as it provides a wide range of functionality that can be used in a variety of situations.
It is a powerful tool that can help developers to create more efficient and robust scripts.
The first method that we'll look at is the argv
attribute.
This attribute is a list that contains the command-line arguments passed to a Python script.
Here's an example of how to use it:
import sys # Print the command-line arguments print(sys.argv)
In this example, we first import the sys module. Then, we use the print()
function to print the sys.argv
attribute, which contains the command-line arguments passed to the script.
Another useful method of the sys module is the exit()
function.
This function is used to exit the interpreter by raising a SystemExit
exception.
The optional argument passed to the function is used as the return code for the script.
Here's an example of how to use it:
import sys # Exit the script with a return code of 1 sys.exit(1)
In this example, we first import the sys module.
Then, we use the exit()
function to exit the script and return a return code of 1.
The version
attribute returns a string that contains the version of the Python interpreter.
Here's an example of how to use it:
import sys # Print the Python version print(sys.version)
In this example, we first import the sys module.
Then, we use the print()
function to print the sys.version
attribute, which contains the version of the Python interpreter.
The path
attribute contains a list of strings that specifies the search path for modules. It can be manipulated to include new paths for importing modules. Here's an example of how to use it:
import sys # Print the module search path print(sys.path) # Add a new path to the search path sys.path.append('/path/to/my/modules') # Print the updated search path print(sys.path)
In this example, we first import the sys module.
Then, we use the print()
function to print the sys.path
attribute, which contains the search path for modules.
Next, we use the append()
method to add a new path to the search path.
Finally, we use the print()
function to print the updated search path.
The sys module also provides some other useful methods and attributes, such as stdin
, stdout
, stderr
, platform
, and exc_info()
that can be used to interact with the interpreter's input and output streams and to get information about the current exception.
In conclusion, the sys module is a powerful module in Python that provides access to various system-specific parameters and functions. It can be used to interact with the underlying operating system, such as interacting with command line arguments and controlling the interpreter's behavior. It is a useful tool for developers to have in their toolkit when working on scripts that need to interact with the system.