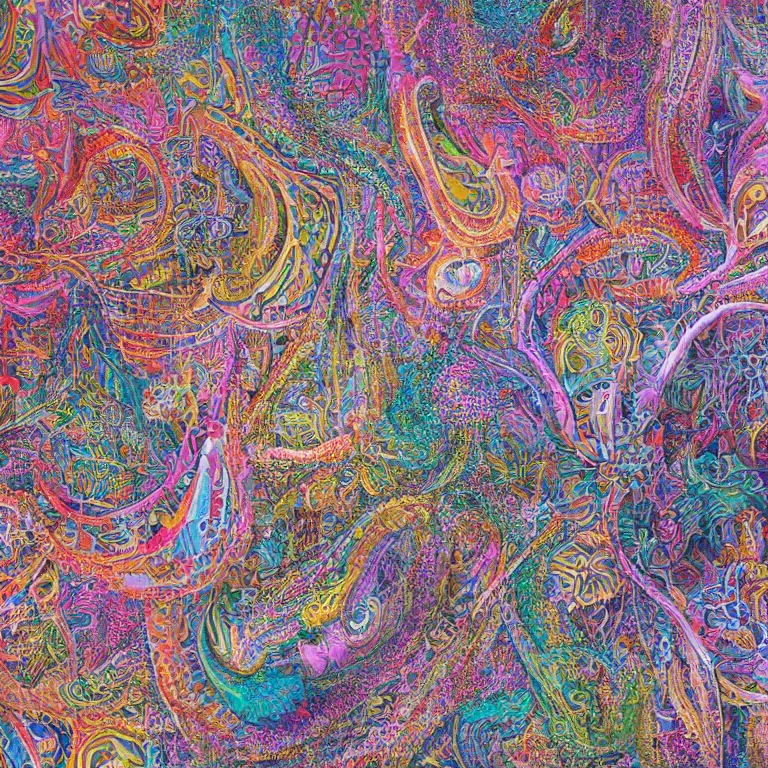
Jan 31st, 2023
The unittest
module is a built-in Python library that allows for the creation and execution of unit tests.
Unit tests are individual tests that check the functionality of specific parts of your code, usually individual functions or methods.
These tests help to ensure that your code is working correctly and that any changes you make in the future won't break any existing functionality.
Many companies require a working knowledge of unit testing for the programming languages they use, and testing is an important part of software engineering.
This post will teach you the basics of unit testing and Python, and this knowledge carries over to most other programming languages that companies use in production code.
Here are some of the most commonly used methods in the unittest
module:
assertEqual(a, b)
: This method checks if the values ofa
andb
are equal. If they are not, it raises anAssertionError
.
import unittest def add(x, y): return x + y class TestAdd(unittest.TestCase): def test_add(self): self.assertEqual(add(3, 4), 7) self.assertEqual(add(-2, 5), 3) self.assertEqual(add(0, 0), 0)
assertTrue(x)
: This method checks if the value ofx
isTrue
. If it is not, it raises anAssertionError
.
import unittest def is_even(x): return x % 2 == 0 class TestIsEven(unittest.TestCase): def test_is_even(self): self.assertTrue(is_even(2)) self.assertTrue(is_even(0)) self.assertTrue(is_even(-4))
assertRaises(exception, function, *args)
: This method checks if the specifiedfunction
raises the specifiedexception
when called with the specifiedargs
. If the function does not raise the exception, it raises anAssertionError
.
import unittest def divide(a, b): return a / b class TestDivide(unittest.TestCase): def test_divide(self): self.assertRaises(ZeroDivisionError, divide, 5, 0)
setUp()
andtearDown()
: These methods are run before and after each test method in the test case, respectively. They can be used to set up any required resources or clean up after a test.
import unittest class TestStringMethods(unittest.TestCase): def setUp(self): self.string = "hello world" def test_upper(self): self.assertEqual(self.string.upper(), "HELLO WORLD") def test_isupper(self): self.assertTrue(self.string.upper().isupper()) def tearDown(self): del self.string
It's important to note that the unittest
library is not the only option for unit testing in Python.
Other popular testing frameworks include pytest
and doctest
.
You can run the unit test by running the python file with the command python -m unittest <file_name>
I hope this gives you a good overview of the unittest
module and its associated methods.
Let me know if you have any questions or need more examples.