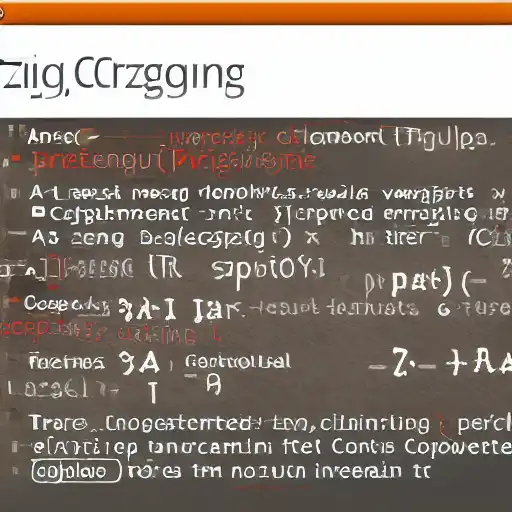
September 9th, 2023
Welcome to the eighth installment of our "Getting Started with Zig on MacOS" series. In this part, we'll delve into the world of error handling in Zig. Zig provides robust mechanisms for dealing with errors, including error sets, error union types, and techniques for handling and propagating errors effectively.
Zig's Error Sets
Error sets are a fundamental feature of Zig's error handling system. They allow you to define a set of errors that a function can return. Error sets provide a way to categorize and document potential issues your code may encounter. Here's how you can define and use an error set:
const std = @import("std"); const ErrorSet = error{ FileNotFound, PermissionDenied, InvalidInput, }; fn openFile(filePath: []const u8) !File { const file = try std.fs.cwd().openFile(filePath, .{}); if (file == null) { return ErrorSet.FileNotFound; } if (file.err != null) { return ErrorSet.PermissionDenied; } return file.ok; }
In this example, we define an error set ErrorSet
with three possible error reasons: FileNotFound
, PermissionDenied
, and InvalidInput
. The openFile
function uses error sets to indicate various error conditions when attempting to open a file.
Error Union Types
Error union types in Zig are used to return either a value or an error from a function. They are declared using the !
symbol. Functions that may return errors are annotated with !
to indicate that they return an error union type. Here's an example:
fn divide(a: i32, b: i32) !f64 { if (b == 0) { return error{ .StandardError = .DivByZero, .name = "Division by zero", }; } return f64(a) / f64(b); }
In this example, the divide
function returns a value of type f64
if the division is successful or an error union type if a division by zero occurs.
Handling and Propagating Errors
To handle errors in Zig, you can use the try
keyword to attempt a potentially error-producing operation and then handle the error using a catch
block. Here's an example of error handling and propagation:
const std = @import("std"); fn main() void { const filePath = "nonexistent.txt"; const result = try openFile(filePath); switch (result) { ErrorSet.FileNotFound => { std.debug.print("File not found: {}\n", .{filePath}); }, ErrorSet.PermissionDenied => { std.debug.print("Permission denied: {}\n", .{filePath}); }, else => { std.debug.print("File opened successfully\n"); // Work with the open file }, } }
In this example, we attempt to open a file using the openFile
function and handle various error conditions using a switch
statement.
What's Next?
In this part, you've learned about Zig's error handling mechanisms, including error sets, error union types, and techniques for handling and propagating errors effectively. Error handling is a critical aspect of writing robust and reliable software.
In Part 9, we'll dive into modules and packages in Zig, helping you organize and structure your code into reusable components and libraries. Stay tuned for more Zig insights and practical examples. Happy coding!