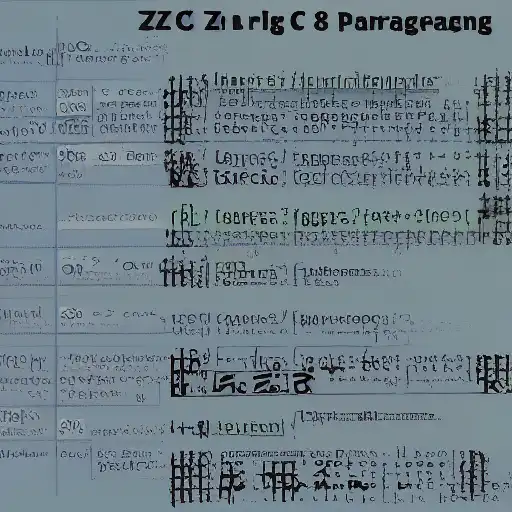
September 8th, 2023
Welcome to the seventh installment of our "Getting Started with Zig on MacOS" series. In this part, we'll dive into the world of pointers and memory management in Zig. Understanding how to work with pointers, allocate and deallocate memory, and navigate Zig's safety features is crucial for writing efficient and reliable code.
Working with Pointers
In Zig, pointers are a way to reference memory locations directly. They allow you to manipulate data and interact with the memory in a low-level manner. Here's an example of declaring and using a pointer in Zig:
const std = @import("std"); fn main() void { const x: i32 = 42; var ptr: *const i32 = &x; std.debug.print("Value at ptr: {}\n", .{@ptr}); }
In this example:
- We declare a variable
x
with the value42
. - We create a pointer
ptr
toptr
using the&
operator. - We use
@ptr
to access the value pointed to byptr
.
Manual Memory Management
Zig provides low-level memory management capabilities, allowing you to allocate and deallocate memory manually when necessary. Here's an example of dynamic memory allocation:
const std = @import("std"); fn main() void { const allocator = std.heap.page_allocator; var ptr: *i32 = try allocator.alloc(i32); defer allocator.free(ptr); ptr.* = 42; std.debug.print("Value at ptr: {}\n", .{ptr.*}); }
In this example:
- We obtain a reference to the heap allocator using
std.heap.page_allocator
. - We allocate memory for an i32 using
allocator.alloc(i32)
. - We use
defer
to ensure that memory is deallocated when we're done with it. - We assign a value to the memory location pointed to by
ptr
.
Safety and the Zig Guarantee
Zig is designed with a strong focus on safety, aiming to eliminate undefined behavior and common programming errors. The Zig compiler performs extensive static analysis to catch issues at compile time rather than runtime. This includes checking for null pointer dereferences, out-of-bounds array accesses, and more.
Here's an example that demonstrates Zig's safety features:
fn main() void { const x: ?i32 = null; const value = if (x) |v| { return v + 1; } else |v| { return 0; }; // This code is safe; Zig ensures that x is not null before dereferencing it. }
In this example, we use the ?
type to indicate that x
can be nullable, and Zig guarantees that we won't dereference a null pointer.
What's Next?
In this part, you've explored pointers, manual memory management, and the safety features of Zig. These concepts are crucial for writing efficient and reliable code in Zig, especially when dealing with low-level programming tasks.
In Part 8, we'll dive deeper into error handling in Zig, including Zig's error sets, error union types, and best practices for handling and propagating errors effectively. Stay tuned for more Zig insights and practical examples. Happy coding!