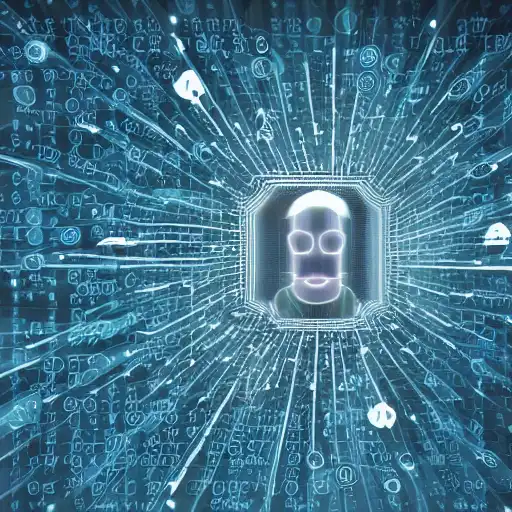
August 10th, 2023
Welcome back to our Advanced Machine Learning series! In this blog post, we'll explore the exciting field of Time Series Analysis, where we'll tackle the challenges and opportunities presented by sequential data.
What is Time Series Analysis?
Time Series Analysis involves the study of data points collected at successive time intervals. These data points are ordered by time, and their temporal relationships contain valuable information that can be leveraged for forecasting, pattern recognition, and anomaly detection.
Key Techniques in Time Series Analysis
- Autoregressive Integrated Moving Average (ARIMA): ARIMA is a classic technique used for time series forecasting. It combines autoregression, differencing, and moving average components to model and predict future values. ARIMA is particularly effective for stationary time series, where the mean and variance do not change over time.
- Seasonal Decomposition of Time Series (STL): STL is used to decompose a time series into three components: seasonal, trend, and residual. By isolating these components, we can gain insights into the underlying patterns and trends, which can be useful for understanding seasonality and making informed decisions.
- Long Short-Term Memory (LSTM) Networks: LSTM networks are a type of recurrent neural network (RNN) designed to handle sequences and long-term dependencies in data. They are particularly effective for modeling non-linear and dynamic patterns in time series, making them popular for tasks like language modeling, speech recognition, and stock market prediction.
Applications of Time Series Analysis
Time Series Analysis finds applications in various domains, including:
- Stock Market Prediction: Time series models can forecast stock prices and assist in making investment decisions.
- Weather Forecasting: Time series techniques are used to predict weather patterns and provide accurate forecasts.
- Healthcare: Time series analysis helps in monitoring patient data, detecting anomalies, and predicting disease outbreaks.
- Manufacturing: Time series models aid in demand forecasting and inventory management.
Implementing Time Series Analysis with Julia
Let's explore how to perform Time Series Analysis with Julia using the ARIMA model and LSTM networks.
# Load required packages using Statistics using DataFrames using Flux using Flux.Data: DataLoader # Load time series data data = CSV.read("time_series_data.csv") time_series = data[:, 2] # Perform seasonal decomposition using STL trend, seasonal, residual = STL(time_series) # Implement ARIMA model model = ARIMA(time_series) # Fit ARIMA model to the data fit!(model) # Forecast future values with ARIMA n_forecast = 12 forecast_arima = forecast(model, n_forecast) # Implement LSTM network for time series forecasting function lstm_forecast(x, h) out, h = LSTM(x, h) return out, h end # Generate sequences for training and testing sequence_length = 10 sequences = [time_series[i:i+sequence_length-1] for i in 1:length(time_series)-sequence_length] x_train, x_test = splitobs(sequences, 0.8) # Convert data to tensors x_train_tensor = [x_train[i] |> Float32 |> gpu for i in 1:length(x_train)] x_test_tensor = [x_test[i] |> Float32 |> gpu for i in 1:length(x_test)] # Train LSTM model model_lstm = Chain(LSTM(1, 10), Dense(10, 1)) loss(x, y) = Flux.mse(model_lstm(x), y) opt = ADAM(0.01) Flux.train!(loss, params(model_lstm), zip(x_train_tensor, x_train_tensor[2:end]), opt) # Forecast future values with LSTM forecast_lstm = [model_lstm(x_train_tensor[end])] |> cpu for _ in 1:n_forecast output = model_lstm(last(forecast_lstm)) push!(forecast_lstm, output) end forecast_lstm = hcat(forecast_lstm...)[:] # Convert forecast to CPU and extract the values forecast_lstm = cpu(forecast_lstm) forecast_lstm = reshape(forecast_lstm, length(forecast_lstm)) # Combine forecasts combined_forecast = [time_series; forecast_arima]
Conclusion
Time Series Analysis is a powerful tool for forecasting, pattern recognition, and decision-making in dynamic environments. In this blog post, we've explored key techniques such as ARIMA and LSTM networks, and implemented Time Series Analysis with Julia.
In the next blog post, we'll dive into the captivating world of Natural Language Processing (NLP), where we'll explore language modeling, sentiment analysis, and other exciting applications of NLP. Stay tuned for more exciting content on our Advanced Machine Learning journey!