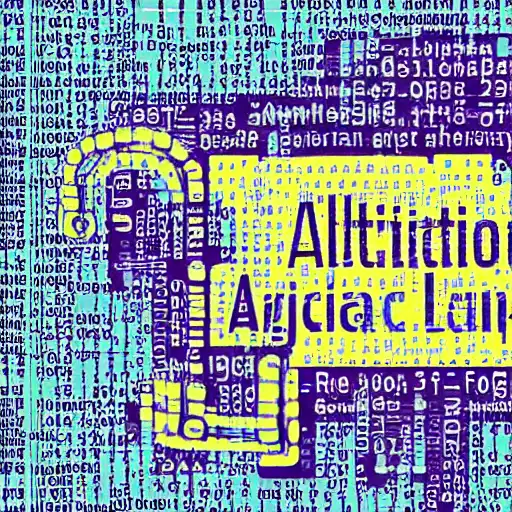
August 9th, 2023
Welcome back to our Advanced Machine Learning series! In this blog post, we'll unveil the secrets of Ensemble Methods, a technique that harnesses the strength of multiple models to boost predictive performance and robustness.
What are Ensemble Methods?
Ensemble Methods combine the predictions of multiple individual models, also known as base learners or weak learners, to make a final prediction. The idea behind Ensemble Methods lies in the principle of "wisdom of the crowd," where the collective intelligence of diverse models can outperform any individual model.
Popular Ensemble Methods
- Bagging (Bootstrap Aggregating): Bagging builds multiple base learners by training each model on different subsets of the training data, selected with replacement. These models vote or average their predictions to make the final decision. Bagging is particularly effective in reducing variance and improving generalization.
- Boosting: Boosting, on the other hand, builds base learners sequentially, where each model focuses on correcting the errors of its predecessors. Boosting assigns weights to the training data, giving more importance to misclassified samples. This iterative process produces a strong ensemble model that performs well on both training and test data.
- Stacking: Stacking combines the predictions of diverse base learners using a meta-model. The base learners are trained on the original training data, and their predictions become the input features for the meta-model. Stacking leverages the complementary strengths of different models to improve overall predictive performance.
Advantages of Ensemble Methods
Ensemble Methods offer several benefits, including:
- Improved Accuracy: Ensemble Methods can achieve higher accuracy compared to individual models, especially when individual models have complementary strengths and weaknesses.
- Robustness: Ensemble Methods tend to be more robust to noisy data and outliers, as the diversity among models helps reduce the impact of individual errors.
- Reduced Overfitting: Bagging and Boosting, in particular, can mitigate overfitting by combining predictions from multiple models.
Implementing Ensemble Methods with Julia
Let's explore how to implement Bagging and Boosting using Julia and Flux.jl.
# Load required packages using Flux using Random # Generate synthetic data function generate_data(n_samples) x = sort(10 * rand(n_samples)) y = 2 * x .+ 5 .+ rand(Normal(0, 2), n_samples) return x, y end x, y = generate_data(100) # Define the base learner (e.g., simple linear regression) base_learner(x, w, b) = w * x .+ b # Define the Bagging ensemble model function bagging_ensemble(x, y, n_models) models = [] for _ in 1:n_models data_subset = sample(1:length(x), length(x), replace = true) w, b = Flux.train!(base_learner, (x[data_subset],), y[data_subset], ADAM(0.01)) push!(models, (w, b)) end return models end # Define the Boosting ensemble model function boosting_ensemble(x, y, n_models) models = [] n_samples = length(x) weights = ones(n_samples) / n_samples for _ in 1:n_models data_subset = sample(1:length(x), length(x), weights = weights, replace = true) w, b = Flux.train!(base_learner, (x[data_subset],), y[data_subset], ADAM(0.01)) predictions = base_learner(x, w, b) errors = abs.(y - predictions) errors_normalized = errors ./ sum(errors) weights = 1 ./ (1 .+ errors_normalized) push!(models, (w, b)) end return models end
Conclusion
Ensemble Methods harness the power of diverse models to achieve superior predictive performance and robustness. In this blog post, we've explored popular Ensemble Methods such as Bagging and Boosting and demonstrated their implementation using Julia and Flux.jl.
In the next blog post, we'll venture into the realm of Time Series Analysis, where we'll tackle the unique challenges posed by sequential data and explore powerful techniques for forecasting and pattern recognition. Stay tuned for more exciting content on our Advanced Machine Learning journey!