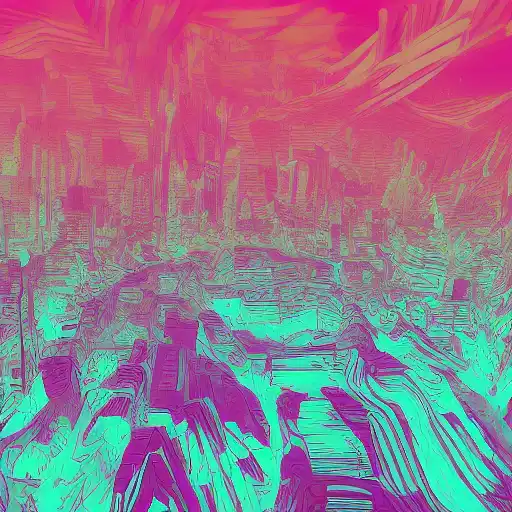
June 10th, 2023
Welcome back to our series on Julia, a powerful and flexible programming language designed for high-performance scientific computing. In the previous post, we discussed setting up a coding environment for Julia on Linux. In this post, we will explore Julia's syntax, unique features, and guide you through writing your first Julia program.
Julia Syntax Basics
Julia's syntax is designed to be easy to read and write, with similarities to other programming languages like Python, MATLAB, and R. Here are some key syntax elements:
Variables and Basic Operations
Assigning values to variables in Julia is simple:
x = 42 y = 3.14
Basic arithmetic operations can be performed using standard symbols:
sum = x + y difference = x - y product = x * y quotient = x / y remainder = x % y
Control Flow
Conditional statements and loops are fundamental to any programming language, and Julia is no exception.
If-elseif-else statements:
x = 42 if x < 0 println("x is negative") elseif x > 0 println("x is positive") else println("x is zero") end
For loops:
for i in 1:5 println(i) end
While loops:
i = 1 while i <= 5 println(i) i += 1 end
Functions
Functions are crucial building blocks of any program. In Julia, you can define functions like this:
function add(x, y) return x + y end result = add(3, 4) # result = 7
Unique Features
Julia has several unique features that set it apart from other programming languages:
- Multiple dispatch: Julia allows you to define function behavior across many combinations of argument types. This enables writing more generic and flexible code.
- Just-In-Time (JIT) compilation: Julia compiles code just before execution, leading to significant performance improvements.
- Metaprogramming: Julia supports metaprogramming, allowing you to create powerful macros and manipulate code as data.
- Interoperability: Julia seamlessly integrates with C and Fortran libraries and can call Python functions using the PyCall package.
Your First Julia Program
Now that you are familiar with Julia's syntax and unique features, let's write a simple program that calculates the factorial of a given number:
function factorial(n) if n == 0 return 1 else return n * factorial(n - 1) end end println("Enter a positive integer:") number = parse(Int, readline()) if number < 0 println("Invalid input. Please enter a positive integer.") else println("The factorial of $number is $(factorial(number))") end
This program defines a recursive function factorial
to compute the factorial of a given number. It then takes input from the user, checks if it's valid, and prints the factorial of the input number.
Conclusion
In this post, we've covered the essentials of Julia programming, including its syntax and unique features. You should now be equipped with the knowledge to write simple Julia programs. In the next post, we will delve into more advanced topics, such as using Julia for data science and machine learning, to further enhance your skills.
Stay tuned and happy coding!