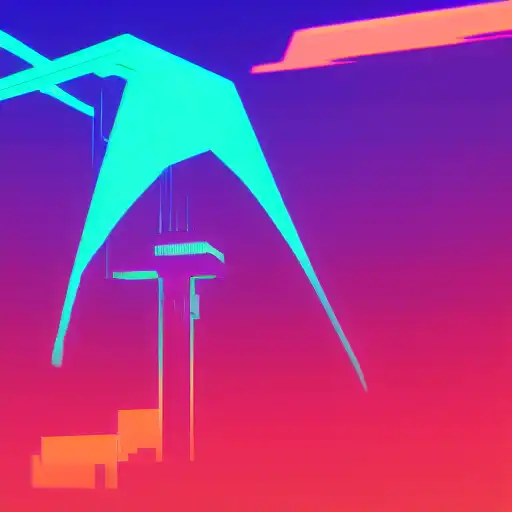
Apr 14th, 2023
In the previous post, we showed you how to add external dependencies to your Java project using Maven. In this post, we'll guide you through the process of creating unit tests for your Java code using JUnit and Maven on Linux.
Step 1: Add the JUnit Dependency to Your pom.xml File
To use JUnit in your Java project, you need to add the JUnit dependency to your pom.xml file. Open the pom.xml file in IntelliJ and add the following lines to the dependencies section:
<dependencies> <dependency> <groupId>junit</groupId> <artifactId>junit</artifactId> <version>4.13.2</version> <scope>test</scope> </dependency> </dependencies>
This will add the JUnit library as a dependency to your project.
Step 2: Create a Java Test Class
In the project explorer, right-click on the "src/test/java" directory and select "New" > "Java Class." Enter the name of your test class, for example, "HelloWorldTest," and click "OK." This will create a new Java test class with a default template.
Step 3: Write Your Test Code
In the editor window, write the following code to create a JUnit test:
import org.junit.Test; import static org.junit.Assert.assertEquals; public class HelloWorldTest { @Test public void testHelloWorld() { HelloWorld helloWorld = new HelloWorld(); String message = helloWorld.getMessage(); assertEquals("Hello, world!", message); } }
This is a simple JUnit test that creates an instance of the HelloWorld class and checks that the message returned by the getMessage() method is "Hello, world!"
Step 4: Build and Run Your Tests
To build and run your tests, open a terminal window and navigate to your project directory. Then, run the following command:
mvn test
This will compile your Java code, download any necessary dependencies, and run your JUnit tests. The output of the tests will be displayed in the terminal window.
Congratulations! You've successfully created unit tests for your Java code using JUnit and Maven on Linux. In the next post, we'll explore how to use Git to manage your Java project.