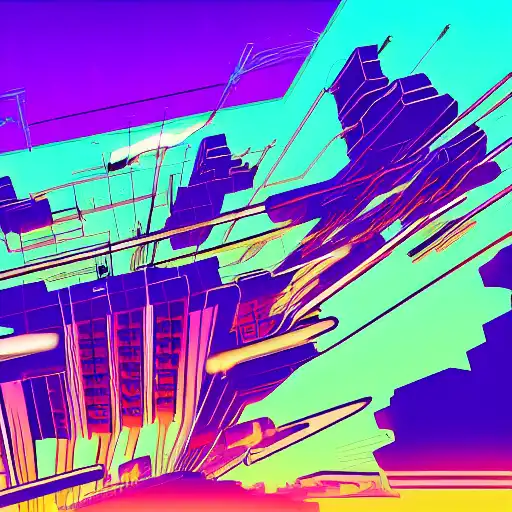
Apr 13th, 2023
In the previous post, we showed you how to create a simple Java application using IntelliJ and Maven on Linux. In this post, we'll guide you through the process of adding external dependencies to your Java project using Maven.
Step 1: Find the Dependency You Need
Before you can add an external dependency to your Java project, you need to find the dependency you need. You can search for dependencies on Maven Central, which is a repository of open-source Java libraries. For example, let's say you want to add the Apache Commons Lang library to your project.
Step 2: Add the Dependency to Your pom.xml File
To add a dependency to your Java project using Maven, you need to modify the pom.xml file in your project directory. Open the pom.xml file in IntelliJ and add the following lines to the dependencies section:
<dependencies> <dependency> <groupId>org.apache.commons</groupId> <artifactId>commons-lang3</artifactId> <version>3.12.0</version> </dependency> </dependencies>
This will add the Apache Commons Lang library as a dependency to your project.
Step 3: Build and Run Your Application
To build your application with the new dependency, open a terminal window and navigate to your project directory. Then, run the following command:
mvn package
This will compile your Java code and download any necessary dependencies. The Apache Commons Lang library will be downloaded to your local repository.
To run your application, use the following command:
java -jar target/myproject.jar
This will run your Java program, which now includes the Apache Commons Lang library.
Congratulations! You've successfully added an external dependency to your Java project using Maven on Linux. In the next post, we'll explore how to create unit tests for your Java code using JUnit and Maven.