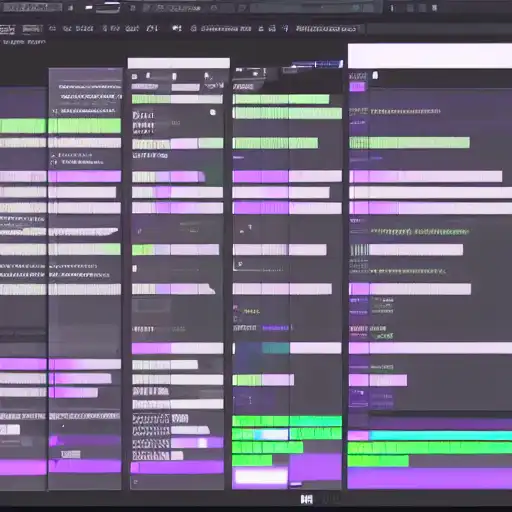
Apr 2nd, 2023
The Iterator Pattern is a behavioral design pattern that provides a way to traverse the elements of a collection without exposing its underlying representation. It is commonly used in programming languages and frameworks to provide a way to iterate over collections of objects.
The pattern involves two main components:
- Iterator: This is an interface that defines methods for accessing and iterating over the elements of a collection. It typically includes methods like hasNext(), next(), and remove().
- Aggregate: This is an interface that defines a method for creating an iterator object. It represents the collection of objects that the iterator will traverse.
Let's take an example of a collection of books in a library. We can use the Iterator Pattern to create an iterator object that allows us to traverse the collection of books without exposing its underlying representation.
First, we define the Iterator interface that includes methods like hasNext(), next(), and remove(). We can then create a Concrete Iterator class that implements the Iterator interface for the collection of books.
Next, we define the Aggregate interface that includes a method for creating an iterator object. We can then create a Concrete Aggregate class that implements the Aggregate interface for the collection of books.
Finally, we can use the iterator object to traverse the collection of books and perform operations on them, such as displaying their titles or checking out a book.
By using the Iterator Pattern, we can create a flexible and extensible system that allows us to traverse collections of objects without exposing their underlying representation. We can also easily create new iterators for different types of collections or modify the behavior of existing iterators.
In conclusion, the Iterator Pattern is a powerful behavioral design pattern that provides a way to traverse the elements of a collection without exposing its underlying representation. By using the pattern, we can create flexible and extensible systems that allow us to iterate over collections of objects and perform operations on them.