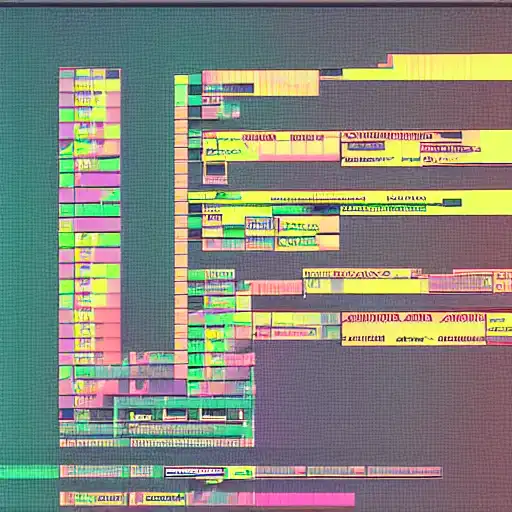
Apr 1st, 2023
The Interpreter Pattern is a behavioral design pattern that defines a grammatical representation for a language and provides an interpreter to interpret sentences in the language. It is commonly used to build compilers and interpreters for programming languages, as well as for implementing domain-specific languages.
The pattern involves three main components:
- Context: This represents the current state of the interpreter and is typically used to store information that is required by the interpreter.
- Abstract Expression: This is an abstract class that represents a grammar rule in the language. It defines an interpret() method that is used to interpret the rule.
- Concrete Expression: These are concrete classes that implement the Abstract Expression class for specific grammar rules in the language.
Let's take an example of a simple programming language that supports basic arithmetic operations, such as addition, subtraction, multiplication, and division. We can implement an interpreter for this language using the Interpreter Pattern.
First, we define the Abstract Expression class that represents a grammar rule for the language, such as an arithmetic expression. We can then create Concrete Expression classes for specific arithmetic operations, such as AdditionExpression, SubtractionExpression, MultiplicationExpression, and DivisionExpression.
Next, we define the Context class that stores the current state of the interpreter, such as the current value of the expression being evaluated. We also create an Interpreter class that uses the Concrete Expression classes to interpret the input expression and perform the corresponding arithmetic operation.
By using the Interpreter Pattern, we can create a flexible and extensible system that can interpret and evaluate expressions in the language. We can also easily add new grammar rules and operations to the language by creating new Concrete Expression classes.
In conclusion, the Interpreter Pattern is a powerful behavioral design pattern that defines a grammatical representation for a language and provides an interpreter to interpret sentences in the language. By using the pattern, we can create flexible and extensible systems that can interpret and evaluate expressions in the language, as well as easily add new grammar rules and operations to the language.