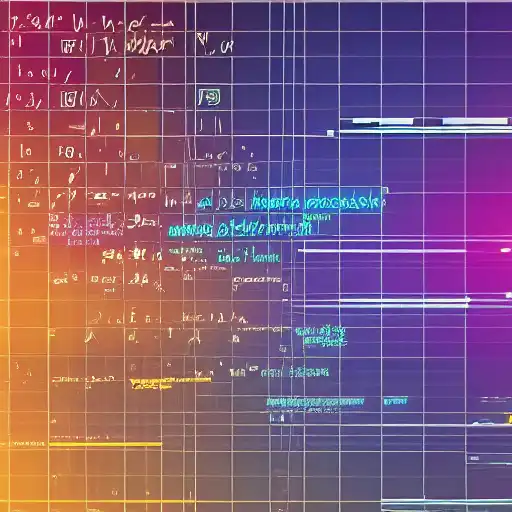
Mar 21st, 2023
Welcome back to our series on design patterns for Object-Oriented Programming (OOP) in Java. In this post, we'll be exploring the Prototype Pattern, which is a creational design pattern that allows us to create new objects by cloning existing objects, instead of creating new objects from scratch.
The Prototype Pattern is used when creating an object is expensive or complicated, and we want to create new objects based on existing ones, but with some modifications. This pattern is commonly used when we need to create a large number of similar objects with small variations.
Let's take a look at the different components of the Prototype Pattern:
-
Prototype Interface:
The Prototype Interface is an interface that defines the methods for cloning the object. This interface is implemented by the concrete prototypes.
-
Concrete Prototype:
The Concrete Prototype is a class that implements the Prototype Interface, and provides a clone() method to create a copy of the object. The concrete prototype is responsible for creating the clone of the object, and making any necessary modifications to the clone.
Now, let's see an example of how we can implement the Prototype Pattern in Java:
public interface Prototype { public Prototype clone(); } public class ConcretePrototype implements Prototype { private String name; public ConcretePrototype(String name) { this.name = name; } public Prototype clone() { return new ConcretePrototype(name); } }
In this example, we have a Prototype interface with a clone() method that is implemented by the ConcretePrototype class. The ConcretePrototype class has a name attribute, and a constructor that takes a name argument. The ConcretePrototype class implements the clone() method by creating a new instance of the ConcretePrototype class with the same name.
Now, let's see how we can use this Prototype pattern:
public class Main { public static void main(String[] args) { ConcretePrototype prototype1 = new ConcretePrototype("Prototype 1"); ConcretePrototype prototype2 = (ConcretePrototype) prototype1.clone(); System.out.println("Prototype 1 name: " + prototype1.getName()); System.out.println("Prototype 2 name: " + prototype2.getName()); prototype2.setName("Prototype 2"); System.out.println("Prototype 1 name: " + prototype1.getName()); System.out.println("Prototype 2 name: " + prototype2.getName()); } }
In this example, we create an instance of the ConcretePrototype class called prototype1. We then create a clone of prototype1 using the clone() method, and store it in prototype2. We print out the names of prototype1 and prototype2, and see that they are the same. We then modify the name of prototype2, and print out the names of prototype1 and prototype2 again. We see that the name of prototype2 has changed, but the name of prototype1 has remained the same.
That's it for our introduction to the Prototype Pattern. We hope you found this post informative and useful. In the next post, we'll explore the Builder Pattern, which is a creational design pattern that separates the construction of a complex object from its representation, allowing us to create different representations using the same construction process. Stay tuned!