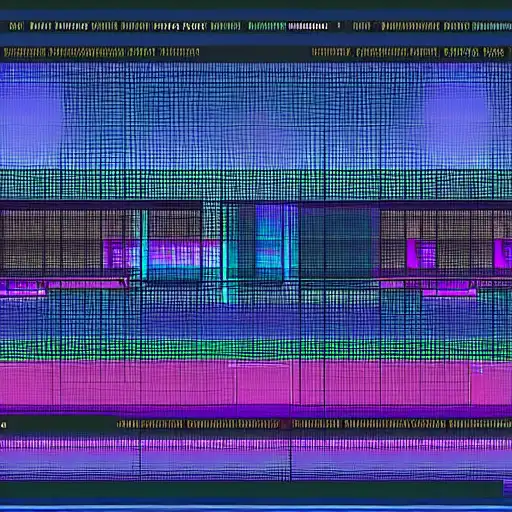
Mar 20th, 2023
Welcome back to our series on design patterns for Object-Oriented Programming (OOP) in Java. In this post, we'll be exploring the Singleton Pattern, which is a creational design pattern that ensures a class has only one instance, and provides a global point of access to it.
The Singleton Pattern is used when we need to ensure that only one instance of a class is created, and provide a way to access that instance from anywhere in our application. This pattern is commonly used in situations where we need to limit the number of instances of a class, such as a configuration manager, a database connection manager, or a logger.
Let's take a look at the different components of the Singleton Pattern:
-
Singleton Class:
The Singleton Class is a class that can have only one instance, and provides a global point of access to that instance. It is responsible for creating and managing the single instance of the class.
-
Private Constructor:
The Private Constructor is a constructor that is made private to prevent the instantiation of the class from outside the class.
-
Instance:
The Instance is a static method that returns the single instance of the class. This method is responsible for creating the instance of the class if it does not exist, and returning the existing instance if it does.
Now, let's see an example of how we can implement the Singleton Pattern in Java:
public class Singleton { private static Singleton instance; private Singleton() { // Private constructor to prevent instantiation from outside the class } public static Singleton getInstance() { if (instance == null) { instance = new Singleton(); } return instance; } }
In this example, we have a Singleton class with a private constructor that prevents the instantiation of the class from outside the class. We also have a static getInstance() method that creates and returns the single instance of the class if it does not exist, and returns the existing instance if it does.
Now, let's see how we can use this Singleton class:
public class Main { public static void main(String[] args) { Singleton singleton1 = Singleton.getInstance(); Singleton singleton2 = Singleton.getInstance(); // singleton1 and singleton2 are the same instance if (singleton1 == singleton2) { System.out.println("singleton1 and singleton2 are the same instance"); } else { System.out.println("singleton1 and singleton2 are not the same instance"); } } }
In this example, we create two instances of the Singleton class using the getInstance() method. We then compare the two instances to see if they are the same instance. Since the Singleton class ensures that only one instance is created, singleton1 and singleton2 are the same instance.
That's it for our introduction to the Singleton Pattern. We hope you found this post informative and useful. In the next post, we'll explore the Prototype Pattern, which is a creational design pattern that allows us to create new objects by cloning existing objects, instead of creating new objects from scratch. Stay tuned!