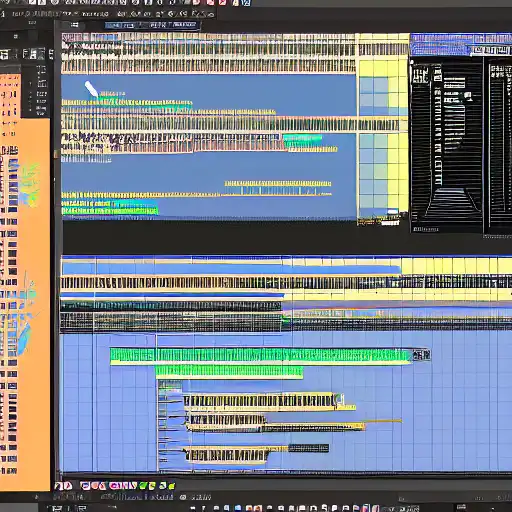
Mar 18th, 2023
Welcome back to our series on design patterns for Object-Oriented Programming (OOP) in Java. In this post, we'll be exploring the Factory Pattern, which is a creational design pattern that provides a way to create objects without specifying their exact class.
The Factory Pattern is used when we need to create objects that are derived from a common base class or interface. It allows us to encapsulate the object creation process and provide a way to create different types of objects based on certain conditions or parameters.
Let's take a look at the different components of the Factory Pattern:
-
Creator:
The Creator is an abstract class or interface that defines a factory method. This method is responsible for creating and returning an object of a certain type. The Creator may also have other methods that use the objects created by the factory method.
-
Concrete Creator:
The Concrete Creator is a subclass of the Creator that implements the factory method. It is responsible for creating and returning a Concrete Product.
-
Product:
The Product is an abstract class or interface that defines the properties and methods that the Concrete Products must have.
-
Concrete Product:
The Concrete Product is a subclass of the Product that implements the properties and methods defined by the Product.
Now, let's see an example of how we can implement the Factory Pattern in Java:
public interface Animal { public void makeSound(); } public class Dog implements Animal { @Override public void makeSound() { System.out.println("Woof"); } } public class Cat implements Animal { @Override public void makeSound() { System.out.println("Meow"); } } public abstract class AnimalFactory { public abstract Animal createAnimal(); } public class DogFactory extends AnimalFactory { @Override public Animal createAnimal() { return new Dog(); } } public class CatFactory extends AnimalFactory { @Override public Animal createAnimal() { return new Cat(); } }
In this example, we have an Animal interface and two Concrete Products, Dog and Cat, that implement this interface. We also have an abstract AnimalFactory class that defines the factory method createAnimal(). Finally, we have two Concrete Creators, DogFactory and CatFactory, that implement the createAnimal() method to create and return the respective Concrete Product.
Now, let's see how we can use these classes to create objects:
public class Main { public static void main(String[] args) { AnimalFactory dogFactory = new DogFactory(); Animal dog = dogFactory.createAnimal(); dog.makeSound(); // Output: Woof AnimalFactory catFactory = new CatFactory(); Animal cat = catFactory.createAnimal(); cat.makeSound(); // Output: Meow } }
In this example, we create a Dog object using the DogFactory and a Cat object using the CatFactory. We then call the makeSound() method on each object to see the respective outputs.
That's it for our introduction to the Factory Pattern. We hope you found this post informative and useful. In the next post, we'll explore the Abstract Factory Pattern, which is a variation of the Factory Pattern that allows us to create families of related objects. Stay tuned!