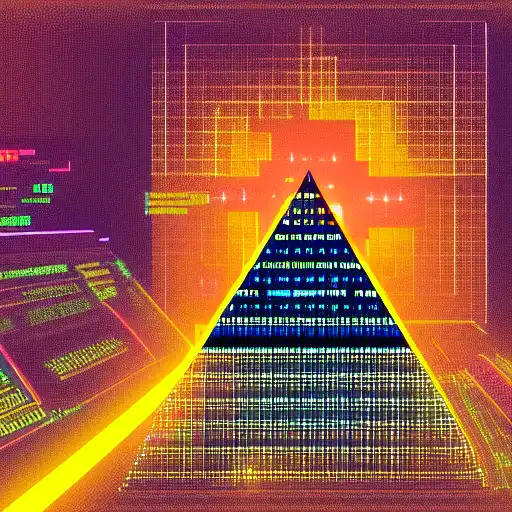
Mar 17th, 2023
Welcome to my new series on design patterns for Object-Oriented Programming (OOP) for Java! In this series, we'll be covering the most commonly used design patterns in OOP, starting with the core Java design patterns.
Java is a popular programming language that is widely used in developing enterprise-level applications. Java has a rich set of libraries and frameworks that support a wide range of design patterns. These design patterns provide solutions to common problems faced in software development, such as creating objects, structuring classes, and managing behaviors.
Java design patterns are classified into three types: Creational, Structural, and Behavioral. Let's take a brief look at each of these types and their sub-parts.
-
Creational Design Pattern:
Creational design patterns deal with object creation mechanisms, trying to create objects in a manner that is suitable for the situation. The five sub-parts of Creational Design Pattern are:
- Factory Pattern: It provides a way to create objects without specifying their exact class.
- Abstract Factory Pattern: It provides an interface for creating families of related or dependent objects without specifying their concrete classes.
- Singleton Pattern: It ensures that only one instance of a class is created and provides a global point of access to it.
- Prototype Pattern: It creates new objects by cloning existing ones.
- Builder Pattern: It separates the construction of a complex object from its representation, allowing the same construction process to create various representations.
-
Structural Design Pattern:
Structural design patterns deal with object composition and structural relationships between them. The seven sub-parts of Structural Design Pattern are:
- Adapter Pattern: It allows incompatible classes to work together by converting the interface of one class into another expected by the clients.
- Bridge Pattern: It decouples an abstraction from its implementation, allowing the two to vary independently.
- Composite Pattern: It composes objects into tree structures to represent part-whole hierarchies.
- Decorator Pattern: It adds new behavior to an object dynamically by wrapping it with a decorator object.
- Facade Pattern: It provides a simplified interface to a complex system of classes, making it easier to use.
- Flyweight Pattern: It minimizes memory usage by sharing data among similar objects.
- Proxy Pattern: It provides a surrogate or placeholder object that controls access to another object.
-
Behavioral Design Pattern:
Behavioral design patterns deal with communication between objects and the assignment of responsibilities between them. The ten sub-parts of Behavioral Design Pattern are:
- Chain Of Responsibility Pattern: It allows multiple objects to handle a request, avoiding coupling the sender of a request to its receiver.
- Command Pattern: It encapsulates a request as an object, allowing it to be passed as a parameter to methods or stored for later use.
- Interpreter Pattern: It defines a grammar for a language and its interpreter that can interpret sentences in the language.
- Iterator Pattern: It provides a way to access the elements of an aggregate object sequentially without exposing its underlying representation.
- Mediator Pattern: It reduces coupling between objects by encapsulating their interactions in a mediator object.
- Memento Pattern: It allows an object to capture its internal state and restore it later.
- Observer Pattern: It defines a one-to-many dependency between objects, so that when one object changes state, all its dependents are notified and updated automatically.
- State Pattern: It allows an object to change its behavior when its internal state changes.
- Strategy Pattern: It defines a family of algorithms, encapsulates each one, and makes them interchangeable.
- Template Pattern: It defines the skeleton of an algorithm in a method, deferring some steps to subclasses.
- Visitor Pattern: It allows adding new operations to an object structure without modifying the objects themselves.
That's a brief overview of the core Java design patterns we'll be covering in this series. Stay tuned for the upcoming posts in which we will dive deeper into each of these design patterns. We'll explore the implementation details, use cases, advantages, and disadvantages of each pattern. By the end of this series, you'll have a strong understanding of the most commonly used design patterns in OOP and be able to apply them in your own projects.
In conclusion, design patterns are a powerful tool for software developers to create reusable and maintainable code. By using design patterns, we can solve common problems in a standard way that is easy to understand and maintain. In this series, we'll focus on the core Java design patterns, but many of these patterns can be applied to other programming languages as well. I hope you'll find this series informative and useful. Stay tuned for the first post on the Factory Pattern!