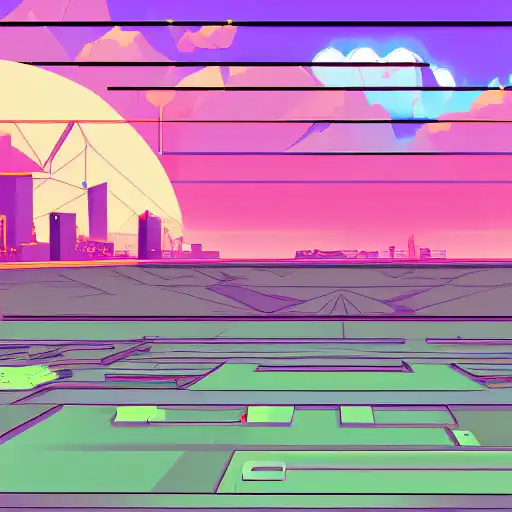
Mar 5th, 2023
Building on our introduction to Racket, in this post, we will explore some of the language's basic features and introduce new topics such as data structures, functions, and control structures. We will also provide some code examples to illustrate these concepts.
Data Structures
Racket provides several built-in data structures, including lists, vectors, and hash tables.
Lists are perhaps the most commonly used data structure in Racket, and they can be created using the list
function:
(define my-list (list 1 2 3))
Lists can be manipulated using a variety of functions, such as cons
, which adds an element to the beginning of a list, and append
, which concatenates two or more lists.
Here's an example that demonstrates these functions:
(define my-list (list 1 2 3)) (define my-other-list (list 4 5 6)) (define my-new-list (append my-list (cons 0 my-other-list)))
In this example, we create two lists (my-list
and my-other-list
), and then we append my-list
to a new list that begins with 0
and then contains my-other-list
.
Functions
Functions are a fundamental concept in Racket, and they are defined using the define
keyword.
Here's an example of a simple function that takes two arguments and returns their sum:
(define (add a b) (+ a b))
This function can be called like this:
(add 1 2) ; returns 3
Functions can also have optional arguments and default values. Here's an example:
(define (multiply a b #:c [c 1]) (* a b c))
This function takes two required arguments (a
and b
) and one optional argument (c
).
If c
is not provided, it defaults to 1
.
The #:c
syntax is used to specify the optional argument.
Control Structures
Racket provides several control structures for controlling the flow of execution in a program.
The most commonly used control structures are if
, cond
, and case
.
Here's an example of an if
statement:
(define (is-positive x) (if (> x 0) #t #f))
This function takes a number x
as an argument and returns #t
if x
is greater than 0
, and #f
otherwise.
Here's an example of a cond
statement:
(define (check-grade grade) (cond [(>= grade 90) "A"] [(>= grade 80) "B"] [(>= grade 70) "C"] [(>= grade 60) "D"] [else "F"]))
This function takes a grade as an argument and returns a letter grade based on the following scale: 90-100 is an A, 80-89 is a B, 70-79 is a C, 60-69 is a D, and anything below 60 is an F.
Conclusion
In this post, we've covered some basic features of Racket, including data structures, functions, and control structures. We've also provided some code examples to illustrate these concepts. In the next post, we will dive deeper into Racket's functional programming capabilities, including higher-order functions and closures.