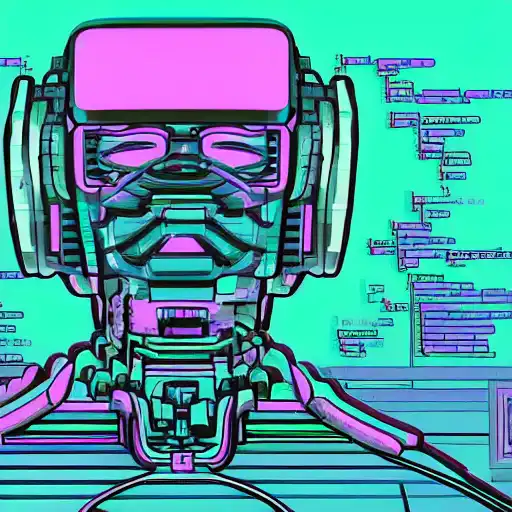
Mar 4th, 2023
Racket is a powerful programming language designed to be a practical and efficient tool for creating software applications, from small scripts to large-scale projects. Its syntax is easy to learn and is similar to that of other Lisp dialects, making it an excellent choice for those familiar with CLIPS, Lisp or Scheme. In this blog post, we will introduce Racket, compare it to other programming languages, discuss its use cases, and provide some coding examples.
Comparison to Other Languages
Racket is a member of the Lisp family of programming languages, which includes Scheme, Common Lisp, and Clojure. Lisp has a reputation for being difficult to learn due to its unusual syntax, but Racket's syntax is relatively easy to understand. Racket is also a functional programming language, which means that it emphasizes immutable data structures and functions that don't have side effects. This makes it a good choice for writing concurrent or parallel programs, as well as for creating software that is less prone to bugs.
Racket is similar to Python in many ways, as both languages prioritize readability and ease of use. However, Racket's type system is more powerful than Python's, allowing for more precise type annotations and better error checking. Racket is also more consistent in its design than Python, as it is built around a small core language with a large number of libraries.
Use Cases
Racket is a versatile language that can be used for a wide range of applications. It is particularly well-suited for creating educational software, such as teaching programming or mathematics. Racket has a built-in teaching language, called Beginning Student Language, that is designed to help beginners learn programming concepts without being overwhelmed by complex syntax. Racket is also used for research in computer science, as its powerful macro system and extensible language design make it a good choice for experimenting with new programming paradigms.
Coding Examples
Let's take a look at some simple examples of Racket code. The following code defines a function that computes the factorial of a number:
(define (factorial n) (if (= n 0) 1 (* n (factorial (- n 1)))))
The next example defines a function that adds two numbers together:
(define (add a b) (+ a b))
As you can see, Racket code is concise and easy to read. Racket also provides a powerful macro system that allows you to define new language constructs. Here is an example of a macro that defines a new loop construct:
(define-syntax-rule (loop i from start to end do body) (for ([i (in-range start end)]) body))
With this macro, you can write loops like this:
(loop i from 1 to 10 do (displayln i))
Conclusion
In conclusion, Racket is a powerful programming language that is versatile, easy to learn, and consistent in its design. It has a wide range of use cases, from educational software to research in computer science. If you're interested in learning Racket, there are plenty of resources available online, including documentation, tutorials, and a supportive community. Give it a try, and you may find that it becomes your new favorite language.