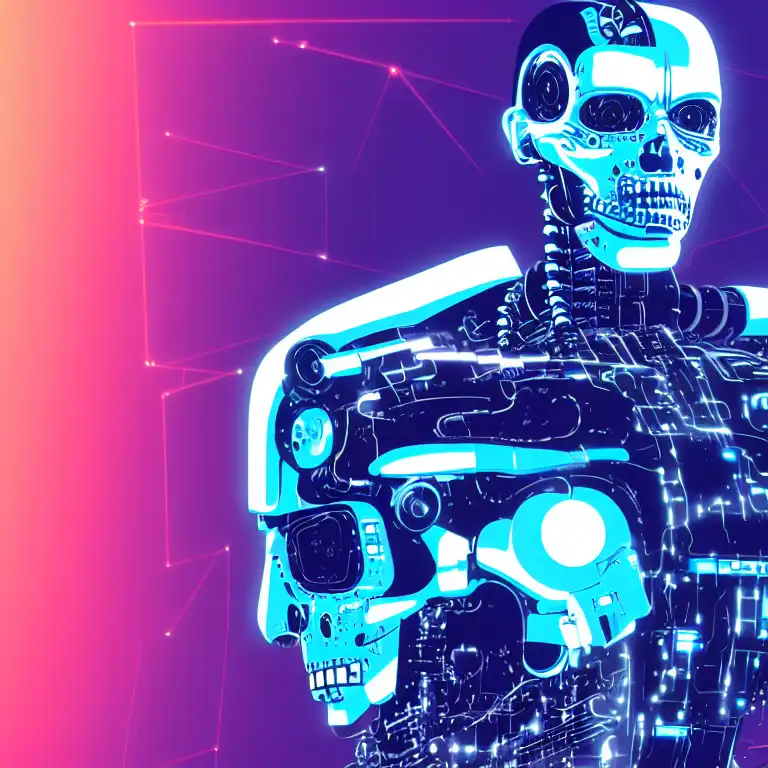
Mar 2nd, 2023
Introduction
Welcome back to the CLIPS series on my professional, personal blog! In this blog post, we will be discussing the topic of using CLIPS connectors for C and C#. CLIPS connectors allow you to integrate CLIPS into other programming languages, such as C and C#. This makes it easier to use CLIPS in your existing projects and take advantage of the benefits of rule-based programming.
Using CLIPS Connector for C
Using the CLIPS connector for C is easy. First, you need to download and install the connector, which can be found on the CLIPS website. After installation, you can use the connector in your C projects by including the header file and linking to the library file.
Here's an example of how you would use the CLIPS connector for C to run a simple rule:
#includeint main() { void *theEnv; theEnv = CreateEnvironment(); /* Load the rules */ Load(theEnv, "my-rules.clp"); /* Define a fact */ AssertString(theEnv, "(my-fact)"); /* Run the rules */ Run(theEnv, -1); /* Destroy the environment */ DestroyEnvironment(theEnv); return 0; }
In this example, the "CreateEnvironment" function is used to create a new CLIPS environment. The "Load" function is used to load the rules from the "my-rules.clp" file. The "AssertString" function is used to define a fact in the environment. The "Run" function is used to run the rules, and the "DestroyEnvironment" function is used to destroy the environment when we are done.
Using CLIPS Connector for C#
Using the CLIPS connector for C# is also easy. First, you need to download and install the connector, which can be found on the CLIPS website. After installation, you can use the connector in your C# projects by referencing the library file and using the CLIPS namespace.
Here's an example of how you would use the CLIPS connector for C# to run a simple rule:
using CLIPSNET; namespace MyProgram { class Program { static void Main(string[] args) { /* Create a new CLIPS environment */ Environment theEnv = new Environment(); /* Load the rules */ theEnv.Load("my-rules.clp"); /* Define a fact */ theEnv.AssertString("(my-fact)"); /* Run the rules */ theEnv.Run(); /* Destroy the environment */ theEnv.Destroy(); } } }
In this example, a new CLIPS environment is created by creating an instance of the "Environment" class. The "Load" method is used to load the rules from the "my-rules.clp" file. The "AssertString" method is used to define a fact in the environment. The "Run" method is used to run the rules, and the "Destroy" method is used to destroy the environment when we are done.
Conclusion
In this blog post, we have discussed the topic of using CLIPS connectors for C and C#. By using the connectors, you can integrate CLIPS into your existing projects and take advantage of the benefits of rule-based programming. The examples provided in this post should give you a good starting point for using the CLIPS connectors in your own projects.
Thank you for following along with the CLIPS series on my blog. Stay tuned for more exciting topics in the future!