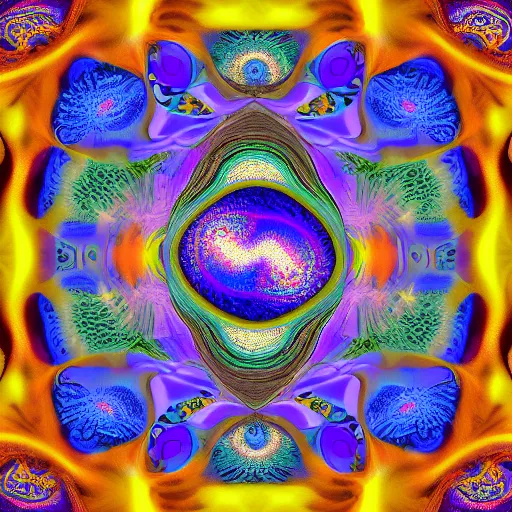
Jan 23rd, 2023
Regular expressions, also known as regex or regexp, are a powerful tool for manipulating and analyzing text.
They are a sequence of characters that define a search pattern, allowing you to match and extract specific information from a string.
You can use the in
operator to check if a specific string is inside a string, but to check if more varied strings are present, you must use regular expressions.
In Python, regular expressions are supported by the re
module, which provides a wide range of functions and methods for working with regular expressions.
The basic syntax of a regular expression in Python is to define a pattern using a string, and then use that pattern to search for matches in another string. The search()
function of the re
module returns a match object if the pattern is found in the string, otherwise it returns None
.
For example:
import re text = "The quick brown fox jumps over the lazy dog" pattern = "fox" match = re.search(pattern, text) if match: print("Found", match.group()) else: print("Not found")
This will output: "Found fox"
You can also use the findall()
function to get a list of all the matches of a pattern in a string, and the finditer()
function to get an iterator of match objects.
For example:
text = "The quick brown fox jumps over the lazy dog" pattern = "o" matches = re.findall(pattern, text) print(matches) # prints ['o', 'o', 'o']
text = "The quick brown fox jumps over the lazy dog" pattern = "o" matches = re.finditer(pattern, text) for match in matches: print(match.group()) # prints 'o' 'o' 'o'
One of the most powerful features of regular expressions is the ability to use special characters and quantifiers to define a pattern.
.
: Matches any character except newline*
: Matches 0 or more of the preceding character+
: Matches 1 or more of the preceding character?
: Matches 0 or 1 of the preceding character{n}
: Matches exactly n occurrences of the preceding character{n,}
: Matches n or more occurrences of the preceding character{n, m}
: Matches between n and m occurrences of the preceding character[]
: A character set. Matches any one of the characters enclosed[^]
: A negated character set. Matches any character not enclosed^
: Matches the start of a string$
: Matches the end of a string()
: Groups a pattern|
: Matches one of the patterns separated by the|
\
: Escapes special characters
For example:
text = "The quick brown fox jumps over the lazy dog" pattern = "^T.+g$" match = re.search(pattern, text) if match: print("Found", match.group()) else: print("Not found")
This will output: "Found The quick brown fox jumps over the lazy dog"
Another important feature of regular expressions is the ability to use special flags to modify the behavior of the pattern. Some of the most commonly used flags are:
re.IGNORECASE
: makes the pattern matching case-insensitivere.MULTILINE
: allows the pattern to match multiple linesre.DOTALL
: makes the dot.
character match any character including newlinere.VERBOSE
: allows you to add comments and white space to the pattern to make it more readable
For example:
text = "The quick brown fox\n jumps over the lazy dog" pattern = "^T.+g$" match = re.search(pattern, text, re.MULTILINE) if match: print("Found", match.group()) else: print("Not found")
This will output: "Found The quick brown fox jumps over the lazy dog"
The re.sub()
method is a function of the re
module in Python, which is used to perform string substitution using regular expressions.
It searches for all occurrences of a pattern in a string and replaces them with a replacement string.
The basic syntax of the re.sub()
method is as follows:
re.sub(pattern, repl, string, count=0, flags=0)
where pattern
is the regular expression pattern to search for, repl
is the replacement string, string
is the target string, count
is the maximum number of occurrences to replace (0 means replace all occurrences), and flags
are any additional regular expression flags.
For example, let's say you have a string with multiple phone numbers in it, but you want to replace them with "xxx-xxx-xxxx" for privacy reasons. You can use the re.sub()
method to do this:
import re text = "My phone number is 555-555-5555 and my friend's number is 666-666-6666" new_text = re.sub(r'\d{3}-\d{3}-\d{4}', 'xxx-xxx-xxxx', text) print(new_text)
This will output: "My phone number is xxx-xxx-xxxx and my friend's number is xxx-xxx-xxxx"
In this example, the re.sub()
method searches for all occurrences of the pattern \d{3}-\d{3}-\d{4}
(which represents a phone number in the format of "xxx-xxx-xxxx") and replaces them with the replacement string "xxx-xxx-xxxx".
The re.sub()
method is a useful tool for performing complex string substitutions, but it should be used with caution as it can easily lead to errors if the regular expression pattern is incorrect.
In such cases it is always recommended to test it with sample inputs before using it in a production environment.
Regular expressions are a powerful tool for working with text in Python, and can be used for tasks such as validating email addresses, parsing logs, or even scraping data from websites. However, it's important to keep in mind that regular expressions can become complex quickly, especially when dealing with large and varied data. It's always a good idea to test and debug your regular expressions with sample data before using them in a production environment.
In conclusion, regular expressions in Python are a powerful tool for working with text, they offer a wide range of special characters and quantifiers, and flags to modify the behavior of the pattern. It is a powerful tool that every python developer should have in their toolbox.
Note: The above examples are just basic examples of regular expressions, you can explore more to get more advanced uses of regular expressions in python. Use the regex 101 website to test out new regular expressions and read deeper into the Pythonic regular expression language to further your knowledge.