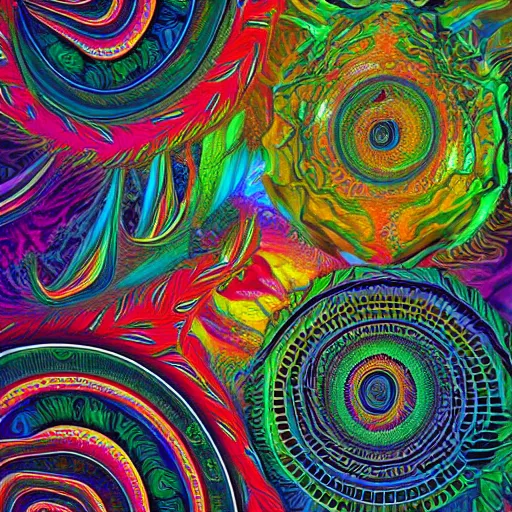
Jan 22nd, 2023
Dictionaries are a fundamental data structure in Python and are used to store key-value pairs in a way that is efficient and easy to manage. Unlike lists and arrays, dictionaries use keys instead of integers to index an element. This allows for a more intuitive and organized way of accessing and manipulating data. Dictionaries are also known as associative arrays, maps, or hash tables in other programming languages. The syntax for creating a dictionary is very simple, you use curly braces {} to enclose a list of key-value pairs, separated by commas. This makes it easy to create and initialize a dictionary with values. The keys in a dictionary must be unique, which makes it easy to avoid duplicate data and ensures that each value can be accessed quickly. The values in a dictionary can be of any data type, including other dictionaries, which allows for complex and nested data structures. The ability to nest dictionaries within each other also opens the possibility for creating more advanced data models. Dictionaries are extremely versatile data structures, and are widely used in many different types of applications, and are an essential part of any Python developer's toolbox.
An example of implementation of a dictionary in Python is as follows:
# create an empty dictionary my_dict = {} # create a dictionary with initial values my_dict = {'name': 'John', 'age': 30, 'city': 'New York'} # create a dictionary using dict() constructor my_dict = dict(name='John', age=30, city='New York')
You can access the value of a dictionary by using its key inside square brackets [], like this:
name = my_dict['name'] print(name) # prints 'John'
You can also use the get()
method to access a value, which is safer than using square brackets because it returns None
if the key is not found, instead of raising a KeyError
:
age = my_dict.get('age') print(age) # prints 30 not_exist = my_dict.get('not_exist') print(not_exist) # prints None
Dictionaries also support several useful methods for adding, modifying, and deleting elements. Some of the most commonly used methods are:
update()
: adds key-value pairs from another dictionary or an iterable of key-value pairs
# adding key-value pairs from another dictionary my_dict.update({'gender': 'male', 'email': 'john@example.com'}) print(my_dict) # prints {'name': 'John', 'age': 30, 'city': 'New York', 'gender': 'male', 'email': 'john@example.com'} # adding key-value pairs from an iterable my_dict.update(zip(['name', 'age'], ['Jane', 25])) print(my_dict) # prints {'name': 'Jane', 'age': 25, 'city': 'New York', 'gender': 'male', 'email': 'john@example.com'}
pop()
: removes and returns a key-value pair by its key
email = my_dict.pop('email') print(email) # prints 'john@example.com' print(my_dict) # prints {'name': 'Jane', 'age': 25, 'city': 'New York', 'gender': 'male'}
popitem()
: removes and returns an arbitrary key-value pair as a tuple
gender = my_dict.popitem() print(gender) # prints ('gender', 'male') print(my_dict) # prints {'name': 'Jane', 'age': 25, 'city': 'New York'}
del
: deletes the key-value pair by its key
del my_dict['city'] print(my_dict) # prints {'name': 'Jane', 'age': 25}
In addition to these methods, dictionaries also have several built-in functions and operators that can be used to manipulate and iterate over the elements. Some of the most commonly used functions and operators are:
len()
: returns the number of key-value pairs in the dictionary
my_dict = {'name': 'John', 'age': 30, 'city': 'New York'} print(len(my_dict)) # prints 3
keys()
andvalues()
: return an iterable of the keys and values respectively
for key in my_dict.keys(): print(key) for value in my_dict.values(): print(value)
items()
: returns an iterable of key-value pairs as tuples
for key, value in my_dict.items(): print(key, value)
in
: checks if a key is present in the dictionary
if 'name' in my_dict: print('name is present') else: print('name is not present')
Dictionaries are a very powerful data structure that can be used for a wide range of tasks, such as storing configuration settings, counting occurrences of words in a text, or creating a cache of frequently used data.
In conclusion, dictionaries in Python are very powerful and versatile data structure, they offer a lot of useful built-in methods and functions that can be used to manipulate and iterate over the elements, making it very flexible to use.
Note: Above examples are just basic examples of dictionaries, you can explore more to get more advanced uses of dictionaries in python.