Jan 20th, 2023
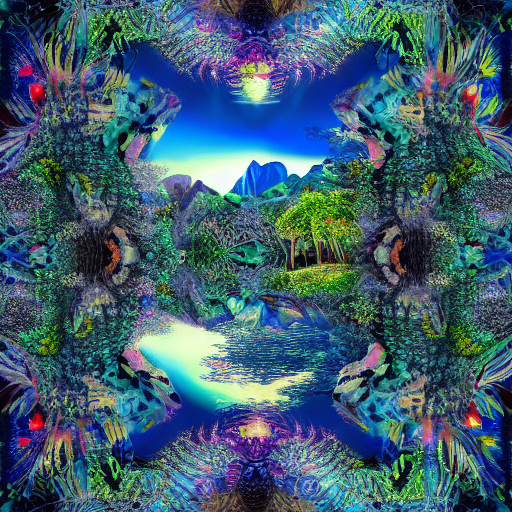
Python is a versatile and powerful programming language, and one of its most basic data types is the string. A string is a sequence of characters, and it can be created by enclosing characters in single quotes ('...') or double quotes ("...").
The way Python implements strings basically is a list of chr
objects, and is used for displaying most text in Python.
You will see strings all over your Python code, and having a solid understanding of the methods and features of strings is essential to your Python knowledge.
String Concatenation
In Python, you can concatenate two or more strings using the +
operator.
For example:
string1 = "Hello" string2 = "World" string3 = string1 + ", " + string2 + "!" print(string3) # Output: "Hello, World!"
String Replication
You can also replicate a string by using the *
operator.
For example:
string = "Python " print(string * 3) # Output: "Python Python Python "
String Slicing
String slicing is a technique used to extract a specific part of a string in Python. It allows you to access individual characters or a range of characters within a string.
In Python, strings are indexed, meaning that each character within a string is assigned a numerical index starting from 0.
To slice a string, you use the []
operator and specify the starting and ending index separated by a colon :
.
The starting index is inclusive, and the ending index is exclusive.
For example, let's say you have the following string:
string = "Python is fun!"
To get the first character of the string, you can use the following code:
print(string[0]) # Output: "P"
To get the last character of the string, you can use the following code:
print(string[-1]) # Output: "!"
To get a range of characters within the string, you can use the following code:
print(string[7:11]) # Output: "is "
You can also use negative indexing to slice the string from the end, for example:
print(string[-5:-1]) # Output: "fun"
You can also leave the starting or ending index blank to slice the string from the beginning or to the end respectively. For example:
print(string[:5]) # Output: "Pytho" print(string[7:]) # Output: "is fun!"
You can also use the step parameter to skip characters while slicing. The step parameter is added after the starting and ending indices and is separated by a colon. For example, to get every second character in a string:
print(string[::2]) # Output: "Pto sfn"
It's important to note that strings are immutable, meaning that once a string is created, it cannot be modified. When you slice a string, you are creating a new string, not modifying the original one.
In summary, string slicing is a powerful technique in Python that allows you to access and manipulate specific parts of a string. It's a versatile and efficient way to work with strings and it's widely used in various applications.
String Methods
Python provides a number of built-in methods for working with strings. Some of the most commonly used methods are:
len()
: Returns the length of a string.str.upper()
: Returns a copy of the string in uppercase.str.lower()
: Returns a copy of the string in lowercase.str.find(sub)
: Returns the index of the first occurrence of the substring.str.replace(old, new)
: Replaces all occurrences of the old substring with the new substring.str.split(sep)
: Returns a list of substrings that are separated by the specified separator.str.strip()
: Removes leading and trailing whitespace from a string.
string = "Python is fun!" print(len(string)) # Output: 14 print(string.upper()) # Output: "PYTHON IS FUN!" print(string.lower()) # Output: "python is fun!" print(string.find("is")) # Output: 7 print(string.replace("is", "was")) # Output: "Python was fun!" print(string.split(" ")) # Output: ['Python', 'is', 'fun!'] string = " Python is fun! " print(string.strip()) # Output: "Python is fun!"
Conclusion
Strings are an essential part of programming, and Python provides a number of built-in methods and operators for working with strings. Whether you're concatenating strings, replicating them, slicing them, or using any of the built-in string methods, it's important to understand the basics of how strings work and how to manipulate them effectively. String slicing is a particularly useful technique for extracting specific parts of a string, and it's often used in combination with other string methods and operations. Understanding how to use string slicing, as well as other string-related features of Python, is a key skill for any programmer. With the knowledge of these techniques, you can work efficiently with strings, making your code more readable, maintainable, and powerful.