Jan 19th, 2023
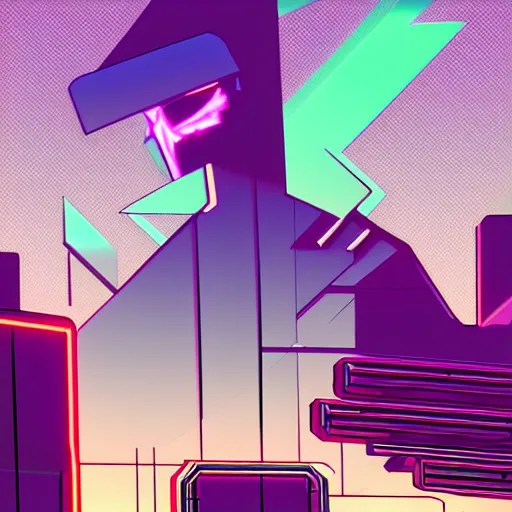
Python is a powerful programming language that is widely used in a variety of applications, from web development and data analysis to machine learning and scientific computing. One area where Python excels is in its ability to work seamlessly with databases, and one of the most popular databases for Python developers is MongoDB. In this post, we'll cover some advanced topics for using the MongoDB module in Python to help you take your skills to the next level.
To start, you'll need to have a MongoDB instance running and accessible from your Python environment. Once you have that set up, you can use the PyMongo library to connect to your MongoDB instance and begin working with your data.
The PyMongo library provides a simple and convenient API for interacting with MongoDB databases.
You can use the MongoClient
class to connect to a MongoDB instance, and the find()
method to retrieve data from a collection.
For example:
from pymongo import MongoClient client = MongoClient('mongodb://localhost:27017/') db = client['mydatabase'] collection = db['mycollection'] data = collection.find() for document in data: print(document)
Another commonly used function in PyMongo is insert_one()
method to insert a single document in a collection.
For example:
from pymongo import MongoClient client = MongoClient('mongodb://localhost:27017/') db = client['mydatabase'] collection = db['mycollection'] my_document = { "name": "John", "age": 30 } collection.insert_one(my_document)
Next, let's look at some more advanced features of the PyMongo library. One of the most powerful features of MongoDB is its ability to handle large amounts of data with high performance and scalability. PyMongo provides several features that make it easy to work with large datasets, including support for aggregation and indexing.
Aggregation allows you to perform complex data processing and analysis on your data, using the MongoDB aggregation pipeline. The aggregation pipeline is a powerful tool that allows you to perform a wide range of operations on your data, such as filtering, grouping, and sorting.
For example, let's say you want to find the average age of all the documents in your collection:
from bson.son import SON pipeline = [ {"$group": {"_id": None, "average_age": {"$avg": "$age"}}} ] result = collection.aggregate(pipeline) print(list(result))
Finally, let's look at how you can use indexing to improve the performance of your MongoDB queries. Indexing allows you to create a special data structure that stores a copy of your data in a specific order, so that it can be retrieved quickly and efficiently. You can create indexes on any field or combination of fields in your documents, and use them to optimize your queries.
For example, let's say you want to create an index on the name field in your collection:
collection.create_index([("name", pymongo.ASCENDING)])
In this post, we've covered some of the advanced topics for using the MongoDB module in Python. We've seen how to connect to a MongoDB instance, retrieve data from a collection, perform complex data processing and analysis with the aggregation pipeline,