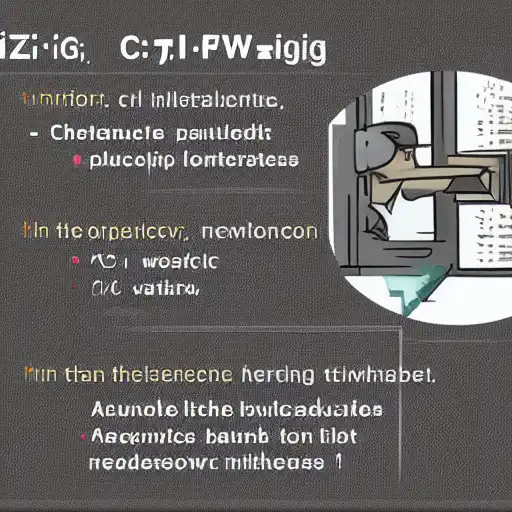
September 11th, 2023
Welcome to the tenth installment of our "Getting Started with Zig on MacOS" series. In this part, we'll dive into unit testing in Zig. Writing tests is a crucial aspect of software development, and Zig provides powerful tools for creating, running, and managing test suites.
Writing Tests in Zig
Zig has built-in support for unit testing through the test
block. To create a test, you define a test
block with a descriptive name and include assertions to validate the behavior of your code. Here's an example of writing a simple test in Zig:
const std = @import("std"); test "Addition test" { const result = my_module.add(3, 4); std.testing.expect(result == 7); }
In this example:
- We create a
test
block with the name "Addition test." - Inside the test block, we call a function from the
my_module
and usestd.testing.expect
to assert that the result is equal to 7.
Running and Managing Test Suites
To run your tests, you can use Zig's built-in test runner. Open your terminal, navigate to your project directory, and run the following command:
zig test my_module.zigThis command will execute all the tests in the specified Zig file (in this case,
my_module.zig
) and display the results in your terminal.
You can organize your tests into multiple files or modules and run them all together using wildcards. For example:
zig test *.zigAdditionally, you can use the
@testable
attribute to make internal functions and variables available for testing. This allows you to test functions that are not exposed in the public API of a module.
Test Coverage and Best Practices
Test coverage is a measure of how much of your code is exercised by your tests. Zig provides tools to measure code coverage to ensure that your tests adequately cover your codebase. You can generate a code coverage report by running your tests with the --test-coverage
flag:
zig test --test-coverage my_module.zigZig also encourages some best practices for writing effective tests:
- Keep your tests focused on specific behaviors or functions.
- Write tests that cover edge cases and boundary conditions.
- Use descriptive test names that convey their purpose.
- Avoid using hardcoded constants in tests; prefer test data generation.
- Regularly run tests to catch regressions and ensure code reliability.
What's Next?
In this part, you've learned how to write unit tests in Zig, run and manage test suites, and measure test coverage. Unit testing is a crucial part of software development, helping you ensure the correctness and reliability of your code.
In our next installment, Part 11, we'll explore Zig's documentation generation tools and best practices for documenting your code. Well-documented code is essential for collaboration and maintainability. Stay tuned for more Zig insights and practical examples. Happy coding!