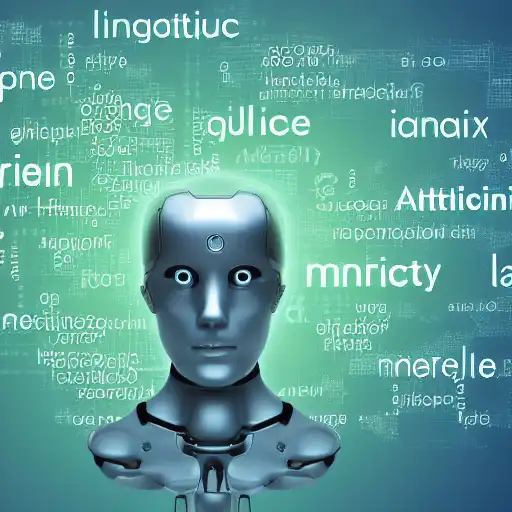
August 17th, 2023
Welcome back to our Advanced Machine Learning series! In this blog post, we'll dive into the captivating world of Reinforcement Learning with Function Approximation, where we combine the strengths of RL and function approximation techniques to tackle complex decision-making tasks.
The Challenge of Complex Decision-Making
In many real-world scenarios, decision-making tasks involve large state and action spaces, making it impractical to represent them with explicit tabular methods. Function Approximation allows us to overcome this challenge by representing value functions or policies with parameterized functions.
Value Function Approximation
Value Function Approximation aims to estimate the value function, which maps states to their expected cumulative rewards. Instead of storing values for all possible states, we use a parameterized function (e.g., a neural network) to approximate the value function.
Deep Q-Learning
Deep Q-Learning is a popular algorithm that combines Q-Learning with function approximation using deep neural networks. The Deep Q-Network (DQN) approximates the Q-value function for each state-action pair, enabling RL agents to handle high-dimensional state spaces.
Experience Replay and Target Networks
To stabilize the training process, DQN uses experience replay and target networks. Experience replay stores transitions from past experiences, and during training, random batches of transitions are used for learning. Target networks help mitigate the problem of moving targets by keeping a separate network with fixed parameters for estimating Q-values during training.
Applications of Reinforcement Learning with Function Approximation
Reinforcement Learning with Function Approximation finds applications in various domains, including:
- Game Playing: RL agents using DQN have achieved remarkable performance in games like Atari and Dota 2.
- Robotics: Function approximation enables robots to make decisions in complex and dynamic environments, facilitating tasks like navigation and manipulation.
- Autonomous Vehicles: RL with function approximation helps self-driving vehicles handle diverse and unpredictable traffic scenarios.
- Finance: RL agents can optimize trading strategies and portfolio management with function approximation.
Implementing Deep Q-Learning with Julia and Flux.jl
Let's explore how to implement Deep Q-Learning with Function Approximation using Julia and Flux.jl.
# Load required packages using Flux using Flux: onehotbatch, mse # Define the Deep Q-Network (DQN) architecture function dqn_model(input_dim, output_dim) return Chain( Dense(input_dim, 64, relu), Dense(64, 32, relu), Dense(32, output_dim) ) end # Initialize the DQN model dqn = dqn_model(input_dim, output_dim) # Define the loss function function dqn_loss(state, action, reward, next_state, done) target = reward + (1 - done) * γ * maximum(dqn(next_state)) q_value = dqn(state)[action] return mse(q_value, target) end # Train the DQN model using Q-Learning and function approximation function train_dqn(env, episodes, batch_size, γ, ε) # Initialize DQN model and optimizer dqn = dqn_model(env.observation_space_dim, env.action_space_dim) opt = ADAM(0.001) for episode in 1:episodes state = env.reset() done = false while !done # Choose action using epsilon-greedy policy if rand() < ε action = rand(1:env.action_space_dim) else q_values = dqn(state) action = argmax(q_values) end # Perform action in the environment next_state, reward, done = env.step(action) # Update the DQN model using Q-Learning with function approximation loss = dqn_loss(state, action, reward, next_state, done) Flux.back!(loss) Flux.update!(opt, dqn) state = next_state end end end
Conclusion
Reinforcement Learning with Function Approximation bridges the gap for complex decision-making tasks, allowing RL agents to handle large state and action spaces effectively. In this blog post, we've explored value function approximation, deep Q-learning, and their applications in game playing, robotics, autonomous vehicles, and finance.
In the next blog post, we'll venture into the realm of Generative Adversarial Networks (GANs) and explore how they can generate realistic data samples with adversarial training. Stay tuned for more exciting content on our Advanced Machine Learning journey!