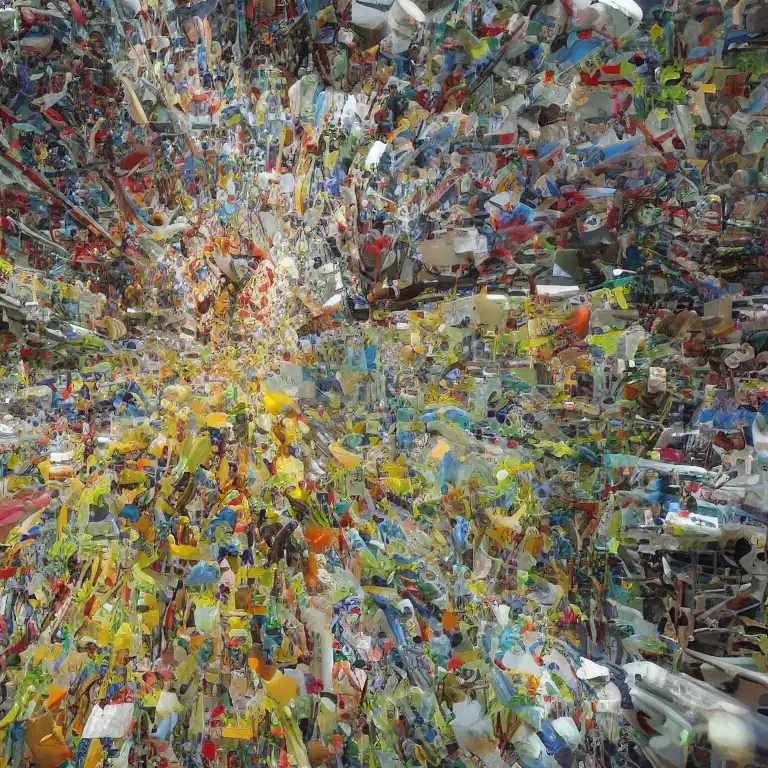
July 19th, 2023
Welcome back to our SQL blog series! In the last post, we covered the basics of SQL and introduced the MySQL database management system. In this post, we will cover how to create a database and table using SQL.
Creating a Database
To create a database using SQL, you can use the CREATE DATABASE command followed by the name of the database. Here's an example:
CREATE DATABASE mydatabase;
This command creates a new database named "mydatabase". You can replace "mydatabase" with the name of your choice.
Creating a Table
Once you have created a database, you can create a table to store data in it. To create a table using SQL, you can use the CREATE TABLE command followed by the name of the table and its columns. Here's an example:
CREATE TABLE mytable ( id INT PRIMARY KEY, name VARCHAR(50), age INT );
This command creates a new table named "mytable" with three columns: "id", "name", and "age". The "id" column is set as the primary key. The "name" column is a variable character (VARCHAR) data type with a maximum length of 50 characters, and the "age" column is an integer (INT) data type.
Inserting Data into a Table
Once you have created a table, you can insert data into it using the INSERT INTO command. Here's an example:
INSERT INTO mytable (id, name, age) VALUES (1, 'John Doe', 25);
This command inserts a new row into the "mytable" table with the values "1" for the "id" column, "John Doe" for the "name" column, and "25" for the "age" column.
Conclusion
In this post, we covered how to create a database and table using SQL. We also covered how to insert data into a table using the INSERT INTO command. In the next post, we will cover how to retrieve data from a table using the SELECT command. Stay tuned!