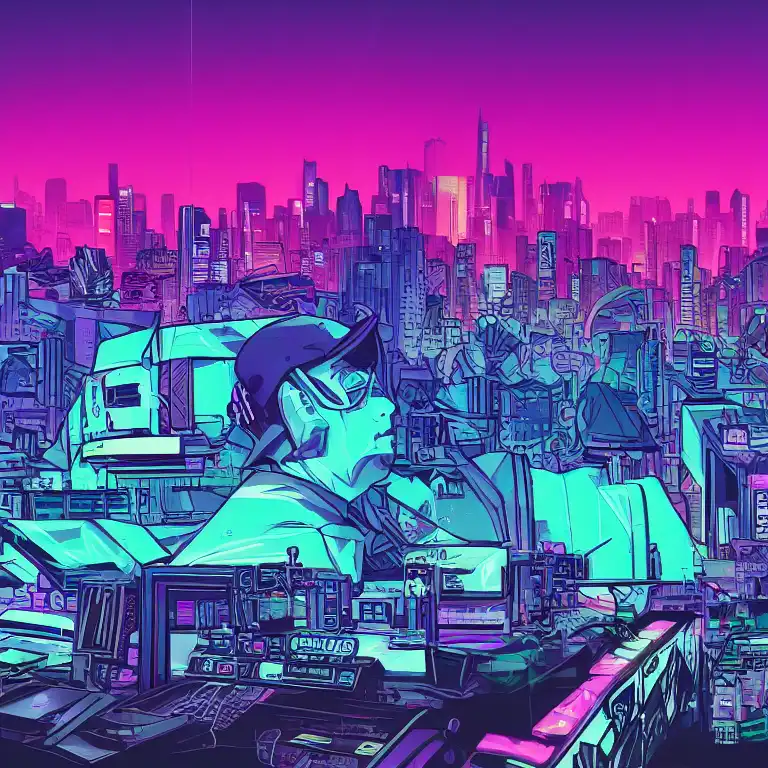
May 29th, 2023
Welcome back to our DevOps series! In the previous posts, we explored how to set up Jenkins, Docker, and Artifactory on a Linux system, and how to use them together to build and test Docker containers, manage and distribute binary artifacts, and create a container registry. In this post, we'll bring it all together and create a complete DevOps pipeline using Jenkins, Docker, and Artifactory.
Step 1: Creating a Jenkins Pipeline
To create a complete DevOps pipeline, we'll use a Jenkins pipeline that includes the following stages:
- Build: Builds the Docker image and tests it
- Publish: Publishes the Docker image to Artifactory
- Deploy: Deploys the Docker container to a production environment
Here's an example Jenkinsfile that includes these stages:
pipeline { agent any stages { stage('Build') { steps { sh 'docker build -t my-image .' sh 'docker run my-image npm test' } } stage('Publish') { steps { script { def server = Artifactory.server 'my-artifactory-server' def buildInfo = Artifactory.newBuildInfo() server.publishBuildInfo(buildInfo) def dockerBuild = Artifactory.dockerBuildInfo() dockerBuild.name = 'my-image' dockerBuild.tag = 'latest' dockerBuild.dockerFile = 'Dockerfile' dockerBuild.contextUrl = '.' dockerBuild.info.add(buildInfo) server.publishDocker(dockerBuild) } } } stage('Deploy') { steps { sshagent(['my-ssh-credentials']) { sh 'ssh user@production-server docker pull ARTIFACTORY_REGISTRY/my-image' sh 'ssh user@production-server docker run -d -p 80:80 ARTIFACTORY_REGISTRY/my-image' } } } } }
This pipeline builds the Docker image, tests it, publishes it to Artifactory, and deploys it to a production environment using SSH.
Step 2: Configuring Production Environment
To deploy the Docker container to a production environment, we'll need to configure the environment with Docker and SSH access.
First, we'll need to install Docker on the production server. This can be done using the package manager of your choice.
Next, we'll need to create an SSH key pair and add the public key to the production server's authorized_keys
file.
Step 3: Running the Pipeline
To run the pipeline, create a new Jenkins job and select "Pipeline" as the job type. In the Pipeline section, select "Pipeline script from SCM" and enter the URL of your Git repository that contains the Jenkinsfile.
Save the job and run it. Jenkins will automatically clone the repository, build and test the Docker container, publish it to Artifactory, and deploy it to the production environment.
And that's it! With this complete DevOps pipeline, we can easily build, test, publish, and deploy Docker containers as part of our software development process. In the final post in this series, we'll wrap up and summarize everything we've learned.