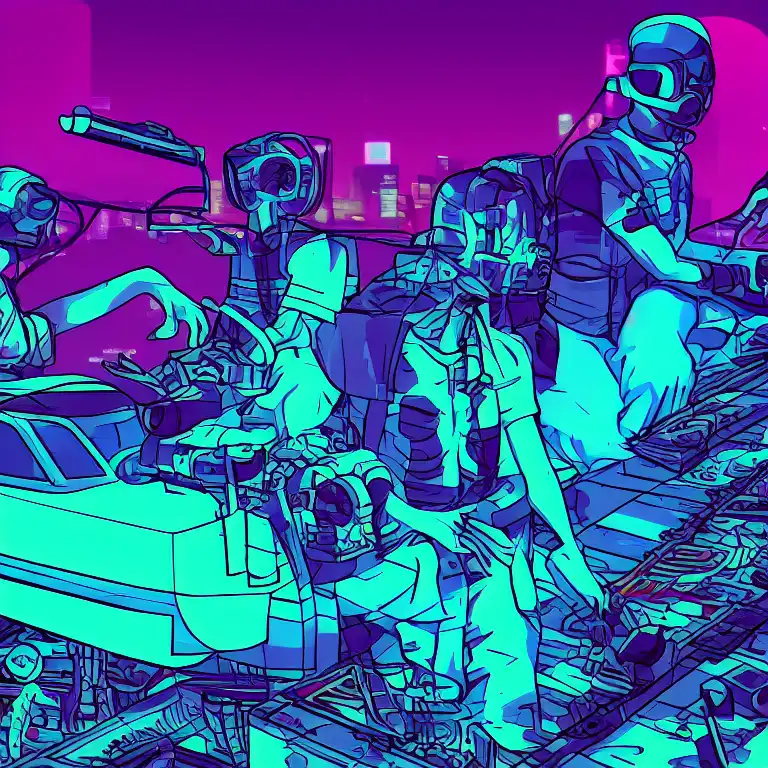
May 26th, 2023
Welcome back to our DevOps series! In the previous post, we set up Jenkins, Docker, and Artifactory on a Linux system. In this post, we'll explore how to set up a Jenkins pipeline to build and test a Docker container and publish it to Artifactory.
Step 1: Creating a Jenkins Pipeline
To create a Jenkins pipeline, we'll use the Jenkinsfile, which is a Groovy script that defines the steps of the pipeline. Here's an example Jenkinsfile that builds a Docker container and tests it:
pipeline { agent any stages { stage('Build') { steps { sh 'docker build -t my-image .' } } stage('Test') { steps { sh 'docker run my-image npm test' } } } }
This pipeline has two stages: Build and Test. In the Build stage, we use the docker build
command to build a Docker image with the tag my-image
. In the Test stage, we use the docker run
command to run the container and run the tests.
Step 2: Configuring Artifactory
Before we can publish the Docker image to Artifactory, we need to configure Jenkins to connect to Artifactory. To do this, we'll need to install the JFrog Artifactory plugin in Jenkins.
Once the plugin is installed, go to Jenkins > Manage Jenkins > Configure System. Scroll down to the Artifactory section and enter the URL of your Artifactory server and the credentials to authenticate with.
Step 3: Publishing to Artifactory
To publish the Docker image to Artifactory, we'll add a new stage to the Jenkinsfile:
pipeline { agent any stages { stage('Build') { steps { sh 'docker build -t my-image .' } } stage('Test') { steps { sh 'docker run my-image npm test' } } stage('Publish') { steps { script { def server = Artifactory.server 'my-artifactory-server' def buildInfo = Artifactory.newBuildInfo() server.publishBuildInfo(buildInfo) def dockerBuild = Artifactory.dockerBuildInfo() dockerBuild.name = 'my-image' dockerBuild.tag = 'latest' dockerBuild.dockerFile = 'Dockerfile' dockerBuild.contextUrl = '.' dockerBuild.info.add(buildInfo) server.publishDocker(dockerBuild) } } } } }
In this stage, we use the Artifactory plugin to create a new build info object and publish it to Artifactory. We then create a new Docker build info object and set the name, tag, Dockerfile, and context URL. Finally, we publish the Docker image to Artifactory using the server.publishDocker
method.
Step 4: Running the Pipeline
To run the pipeline, create a new Jenkins job and select "Pipeline" as the job type. In the Pipeline section, select "Pipeline script from SCM" and enter the URL of your Git repository that contains the Jenkinsfile.
Save the job and run it. Jenkins will automatically clone the repository, build and test the Docker container, and publish it to Artifactory.
And that's it! With this pipeline in place, you can easily build, test, and publish Docker containers to Artifactory as part of your software development process. In the next post in this series, we'll explore how to use Artifactory to manage and distribute binary artifacts.