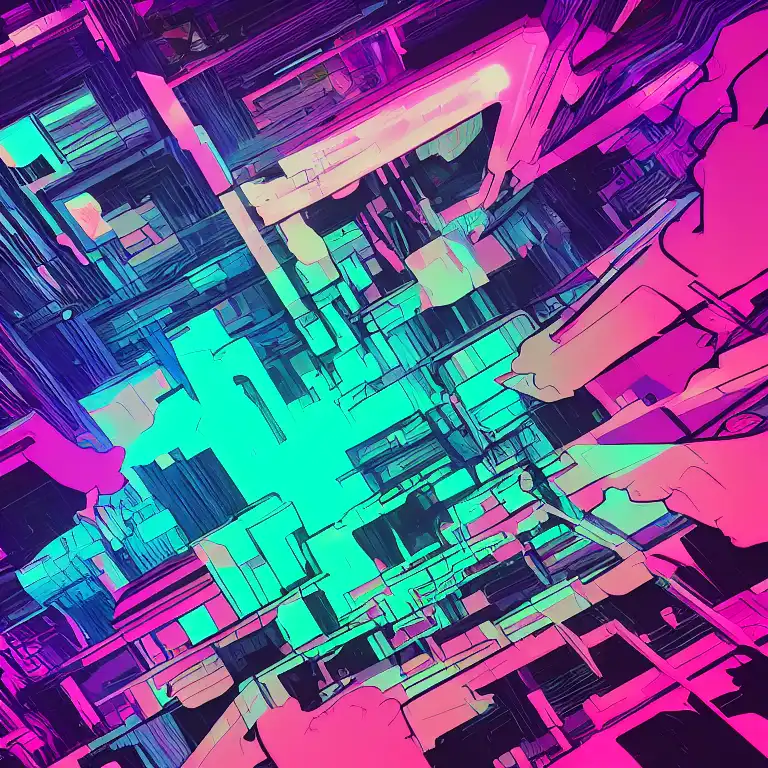
May 16th, 2023
In this post, we will explore how to add authentication and authorization to your web application using ASP.NET Core Identity.
Step 1: Understanding ASP.NET Core Identity
ASP.NET Core Identity is a membership system that allows you to add authentication and authorization to your web applications. It provides a set of libraries and tools for managing user authentication, roles, and permissions.
Some of the key features of ASP.NET Core Identity include:
- User Registration and Management: You can register new users and manage user accounts, including password resets and account lockouts.
- Authentication: You can authenticate users using a variety of methods, such as cookies, tokens, or external providers like Google or Facebook.
- Authorization: You can define roles and permissions for your application and control access to specific areas or features.
- Two-Factor Authentication: You can enable two-factor authentication for added security.
- Social Login: You can allow users to sign in with their social media accounts.
Step 2: Adding Authentication and Authorization to Your Web Application
To add authentication and authorization to your web application using ASP.NET Core Identity, follow these steps:
- Install the ASP.NET Core Identity package. In Visual Studio, right-click on your project and select "Manage NuGet Packages". Search for "Microsoft.AspNetCore.Identity" and install the package.
- Update your DbContext. Open your DbContext file and add the following code:
using Microsoft.AspNetCore.Identity.EntityFrameworkCore; using Microsoft.EntityFrameworkCore; public class ApplicationDbContext : IdentityDbContext { public ApplicationDbContext(DbContextOptions<ApplicationDbContext> options) : base(options) { } }
- Configure ASP.NET Core Identity. In the "Startup.cs" file, add the following code to the "ConfigureServices" method:
services.AddDbContext<ApplicationDbContext>(options => options.UseSqlServer(Configuration.GetConnectionString("DefaultConnection"))); services.AddIdentity<IdentityUser, IdentityRole>() .AddEntityFrameworkStores<ApplicationDbContext>() .AddDefaultTokenProviders(); services.Configure<IdentityOptions>(options => { // Password settings options.Password.RequireDigit = true; options.Password.RequireLowercase = true; options.Password.RequireNonAlphanumeric = true; options.Password.RequireUppercase = true; options.Password.RequiredLength = 8; options.Password.RequiredUniqueChars = 1; // Lockout settings options.Lockout.DefaultLockoutTimeSpan = TimeSpan.FromMinutes(30); options.Lockout.MaxFailedAccessAttempts = 5; options.Lockout.AllowedForNewUsers = true; // User settings options.User.AllowedUserNameCharacters = "abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ0123456789-._@+"; options.User.RequireUniqueEmail = true; // SignIn settings options.SignIn.RequireConfirmedEmail = false; options.SignIn.RequireConfirmedPhoneNumber = false; });
- Add authentication middleware. In the "Startup.cs" file, add the following code to the "Configure" method:
app.UseAuthentication();
- Add authorization middleware. In the "Startup.cs" file, add the following code to the "Configure" method:
app.UseAuthorization();
- Create a user. You can create a user using the UserManager class. For example, to create a new user, you can add the following code to your controller:
var user = new IdentityUser { UserName = "testuser", Email = "testuser@example.com" }; var result = await _userManager.CreateAsync(user, "P@ssw0rd");
- Protect a route. To protect a route, you can add the [Authorize] attribute to your controller or action method. For example:
[Authorize] public IActionResult Secret() { return View(); }
Congratulations! You have successfully added authentication and authorization to your web application using ASP.NET Core Identity and C#.
In this series, we covered the basics of C# programming, how to set up a coding environment on Windows, how to create a simple web application using ASP, how to deploy your web application to a hosting provider, and how to add authentication and authorization using ASP.NET Core Identity.
ASP.NET Core is a powerful framework for building web applications using C#. It provides a wide range of tools and libraries for web development, making it easy to build scalable, maintainable, and secure applications.
We hope this series has helped you get started with C# web programming using ASP.NET Core. If you have any questions or feedback, feel free to leave a comment below. Happy coding!