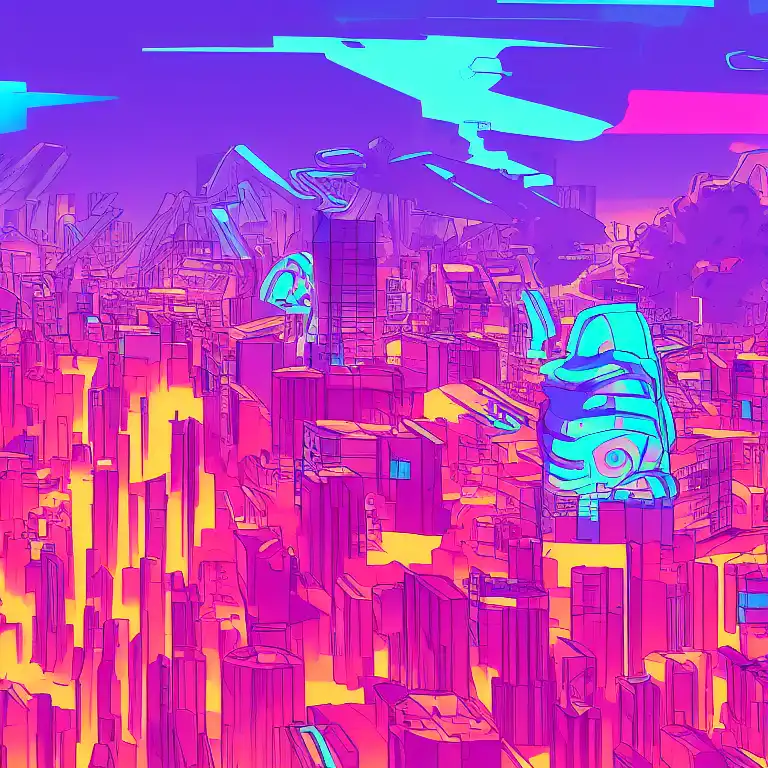
May 14th, 2023
In this post, we will explore how to add more functionality to our web application using C#.
Step 1: Understanding ASP.NET Core
ASP.NET Core is a modern, open-source framework for building web applications using C#. It is designed to be fast, modular, and cross-platform, meaning you can develop and deploy ASP.NET Core applications on Windows, Linux, and macOS.
Some of the key features of ASP.NET Core include:
- Razor Pages: A lightweight, easy-to-use framework for building web pages using C# and HTML.
- MVC: A powerful framework for building scalable, maintainable web applications using the Model-View-Controller (MVC) pattern.
- Web APIs: A framework for building RESTful APIs using C# and JSON.
- Middleware: A set of components that can be added to an ASP.NET Core application to handle cross-cutting concerns such as authentication, caching, and logging.
Step 2: Adding Functionality to our Web Application
Let's add some functionality to our web application. In this example, we will add a form that allows users to add new items to a list.
- Create a new model class. Right-click on the "Models" folder and select "Add" > "Class". Name the class "Item" and add the following properties:
public class Item { public int Id { get; set; } public string Name { get; set; } public string Description { get; set; } }
- Create a new controller. Right-click on the "Controllers" folder and select "Add" > "Controller". Name the controller "ItemController". Add the following code to the controller:
public class ItemController : Controller { private readonly List- _items = new List
- (); public IActionResult Index() { return View(_items); } [HttpGet] public IActionResult Create() { return View(); } [HttpPost] public IActionResult Create(Item item) { item.Id = _items.Count + 1; _items.Add(item); return RedirectToAction("Index"); } }
- Create a new view. Right-click on the "Views" folder and select "Add" > "Folder". Name the folder "Item". Right-click on the "Item" folder and select "Add" > "View". Name the view "Create". Add the following code to the view:
@model Item <form asp-action="Create" method="post"> <div> <label asp-for="Name"></label> <input asp-for="Name" /> </div> <div> <label asp-for="Description"></label> <textarea asp-for="Description"></textarea> </div> <button type="submit">Add Item</button> </form>
- Update the "Index" view. Open the "Index.cshtml" file in the "Views/Item" folder and add the following code:
<a asp-action="Create">Add Item</a> <ul> @foreach (var item in Model) { <li>@item.Name - @item.Description</li> } </ul>
Congratulations! You have added functionality to your web application using C# and ASP.NET Core. In the next post, we will explore how to deploy your web application to a hosting provider.