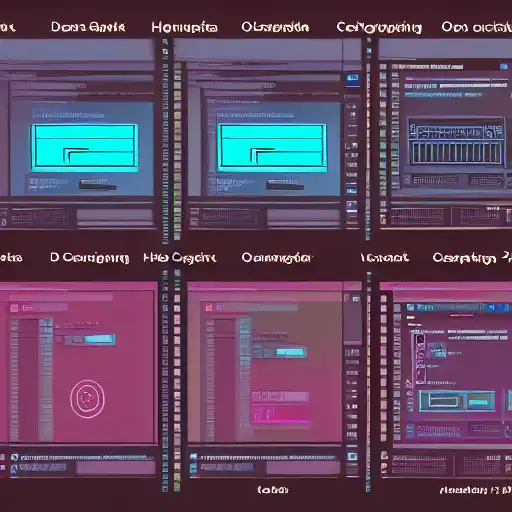
Apr 8th, 2023
The Template pattern is a behavioral pattern that defines the skeleton of an algorithm in a superclass but allows subclasses to override specific steps of the algorithm without changing its structure. This pattern is used to define the steps of an algorithm once and let the subclasses provide the implementation of certain steps.
The Template pattern consists of two main parts: an abstract class that defines the template method, and concrete classes that implement the specific steps of the algorithm. The template method is declared as final to prevent subclasses from changing the order of the steps.
Let's take a look at an example of how to use the Template pattern in C#.
using System; // Abstract class that defines the template method abstract class AbstractClass { public void TemplateMethod() { Step1(); Step2(); Step3(); } public abstract void Step1(); public abstract void Step2(); public void Step3() { Console.WriteLine("AbstractClass: Step 3"); } } // Concrete class that implements specific steps of the algorithm class ConcreteClassA : AbstractClass { public override void Step1() { Console.WriteLine("ConcreteClassA: Step 1"); } public override void Step2() { Console.WriteLine("ConcreteClassA: Step 2"); } } // Concrete class that implements specific steps of the algorithm class ConcreteClassB : AbstractClass { public override void Step1() { Console.WriteLine("ConcreteClassB: Step 1"); } public override void Step2() { Console.WriteLine("ConcreteClassB: Step 2"); } } // Client code class Client { static void Main(string[] args) { AbstractClass obj1 = new ConcreteClassA(); obj1.TemplateMethod(); AbstractClass obj2 = new ConcreteClassB(); obj2.TemplateMethod(); } }
In the above code, we define an abstract class AbstractClass
that declares the template method TemplateMethod()
. The TemplateMethod()
consists of three steps: Step1()
, Step2()
, and Step3()
. Step1()
and Step2()
are abstract methods that are implemented by the concrete classes ConcreteClassA
and ConcreteClassB
.
We then define two concrete classes that implement Step1()
and Step2()
differently. Finally, we create instances of these classes and call their TemplateMethod()
method to execute the algorithm.
By using the Template pattern, we can define the basic structure of an algorithm in the abstract class and allow subclasses to override specific steps without changing the overall structure. This promotes code reuse and makes the code more maintainable.
I hope you found this post informative and helpful. In the next post, we will be discussing the Visitor pattern, which is another behavioral pattern that allows us to separate an algorithm from the object structure on which it operates. Stay tuned!