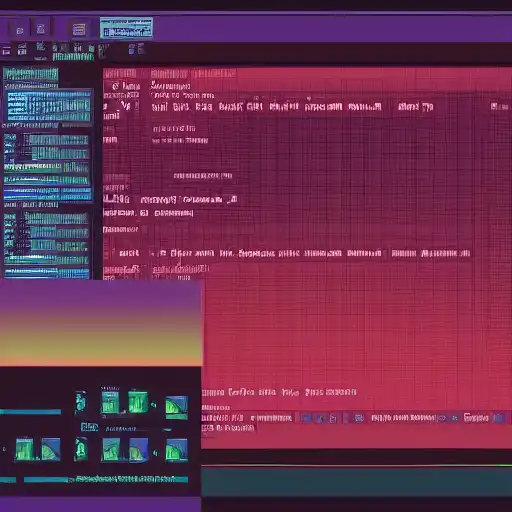
Apr 5th, 2023
The Observer Pattern is a behavioral design pattern that allows objects to subscribe to an event and receive notifications when that event occurs. This pattern is commonly used in applications where there is a need to keep multiple objects updated about the state of a particular object or system.
In this pattern, there are two main roles: the Subject and the Observer. The Subject is the object that is being observed and maintains a list of all the Observer objects that have subscribed to it. When the Subject's state changes, it notifies all the Observer objects by calling a method on each of them. The Observer objects then update themselves based on the new state of the Subject.
The Observer Pattern has several advantages. First, it enables loose coupling between the Subject and Observer objects, making it easy to add new Observers or modify the behavior of existing Observers without affecting the Subject. Second, it allows for a one-to-many relationship between the Subject and Observer objects, enabling efficient broadcasting of updates to multiple Observers. Finally, it supports the Open/Closed Principle, allowing new Observers to be added without modifying existing code.
To implement the Observer Pattern in Java, you can follow these steps:
- Define the Subject interface: This interface defines the methods that will be used to manage the list of Observers and to notify them when the Subject's state changes.
- Define the Observer interface: This interface defines the method that will be called by the Subject when its state changes.
- Implement the Subject class: This class implements the Subject interface and maintains the list of Observers that have subscribed to it. It also provides methods to add and remove Observers from the list.
- Implement the Observer class(es): These classes implement the Observer interface and provide the logic for updating themselves based on the Subject's state.
Here's an example implementation of the Observer Pattern in Java:
// Step 1: Define the Subject interface public interface Subject { public void attach(Observer observer); public void detach(Observer observer); public void notifyObservers(); } // Step 2: Define the Observer interface public interface Observer { public void update(); } // Step 3: Implement the Subject class public class ConcreteSubject implements Subject { private List<Observer> observers = new ArrayList<>(); private String state; public void attach(Observer observer) { observers.add(observer); } public void detach(Observer observer) { observers.remove(observer); } public void notifyObservers() { for (Observer observer : observers) { observer.update(); } } public void setState(String state) { this.state = state; notifyObservers(); } public String getState() { return state; } } // Step 4: Implement the Observer class(es) public class ConcreteObserver implements Observer { private String observerState; private ConcreteSubject subject; public ConcreteObserver(ConcreteSubject subject) { this.subject = subject; } public void update() { observerState = subject.getState(); System.out.println("Observer state updated to: " + observerState); } }
In this example, the ConcreteSubject class is the Subject and the ConcreteObserver class is the Observer. When the ConcreteSubject's state changes, it calls the notifyObservers() method, which in turn calls the update() method on each of the Observers. The ConcreteObserver then updates itself based on the new state of the ConcreteSubject.
Overall, the Observer Pattern is a useful pattern for implementing event-driven architectures and for keeping multiple objects in sync with each other.