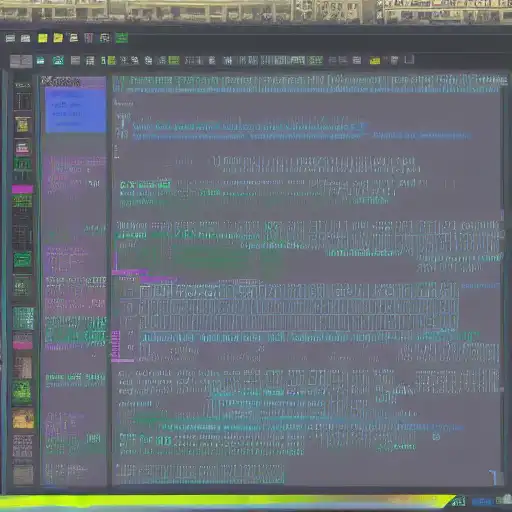
Mar 25th, 2023
The Composite Pattern is a structural design pattern that allows us to treat individual objects and groups of objects uniformly. It lets us create a tree-like structure of objects and manipulate them as a single object.
The Composite Pattern consists of three main components: the Component
, Leaf
, and Composite
. The Component
is the abstract class that defines the interface for all the objects in the tree. The Leaf
is the class that represents the individual objects in the tree. The Composite
is the class that represents the group of objects in the tree.
Let's take an example of a file system. A file system is a tree-like structure that consists of directories and files. Directories can contain other directories and files. Files cannot contain anything else. We can use the Composite Pattern to represent the file system as a tree structure.
We can define our Component
as an abstract class called FileSystemComponent
. It will have two abstract methods, display()
and size()
, that will be implemented by the Leaf
and Composite
classes.
The Leaf
class will be the File
class, which will represent an individual file in the file system. It will implement the FileSystemComponent
abstract class and provide its own implementation of the display()
and size()
methods.
The Composite
class will be the Directory
class, which will represent a group of files and directories in the file system. It will implement the FileSystemComponent
abstract class and have a collection of FileSystemComponent
objects to represent the files and directories it contains. It will also provide its own implementation of the display()
and size()
methods, which will iterate over its collection of FileSystemComponent
objects and call their respective display()
and size()
methods.
The Client
class will create the file system tree by creating Directory
and File
objects and adding them to each other. It will also call the display()
and size()
methods on the root Directory
object to display and calculate the size of the entire file system tree.
By using the Composite Pattern, we can treat individual files and groups of files uniformly, which makes it easier to manipulate and traverse the file system tree. We can also add new types of FileSystemComponent
objects without affecting the existing code, making it easier to extend and modify the file system structure.
In conclusion, the Composite Pattern is a powerful structural design pattern that allows us to create a tree-like structure of objects and manipulate them as a single object. By using the Composite Pattern, we can improve the flexibility and maintainability of our code. I hope this has helped you see the usefulness of the Composite Pattern and will see you in the next post!